Note
Go to the end to download the full example code.
Compares dot implementations (numpy, cython, c++, sse)¶
numpy has a very fast implementation of the dot product. It is difficult to be better and very easy to be slower. This example looks into a couple of slower implementations with cython. The tested functions are the following:
import numpy
import matplotlib.pyplot as plt
from pandas import DataFrame, concat
from teachcompute.validation.cython.dot_cython import (
dot_product,
ddot_cython_array,
ddot_cython_array_optim,
ddot_array,
ddot_array_16,
ddot_array_16_sse,
)
from teachcompute.validation.cython.dot_cython import (
sdot_cython_array,
sdot_cython_array_optim,
sdot_array,
sdot_array_16,
sdot_array_16_sse,
)
from teachcompute.ext_test_case import measure_time_dim
def get_vectors(fct, n, h=100, dtype=numpy.float64):
ctxs = [
dict(
va=numpy.random.randn(n).astype(dtype),
vb=numpy.random.randn(n).astype(dtype),
dot=fct,
x_name=n,
)
for n in range(10, n, h)
]
return ctxs
numpy dot¶
0%| | 0/100 [00:00<?, ?it/s]
77%|███████▋ | 77/100 [00:00<00:00, 763.11it/s]
100%|██████████| 100/100 [00:00<00:00, 730.58it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000003 9.925625e-08 0.000002 0.000003 10 50 0.000025 184 0.000011 9710 numpy.dot
98 0.000003 9.436711e-09 0.000003 0.000003 10 50 0.000025 184 0.000012 9810 numpy.dot
99 0.000003 1.160821e-07 0.000003 0.000003 10 50 0.000026 184 0.000010 9910 numpy.dot
Several cython dot¶
for fct in [
dot_product,
ddot_cython_array,
ddot_cython_array_optim,
ddot_array,
ddot_array_16,
ddot_array_16_sse,
]:
ctxs = get_vectors(fct, 10000 if fct.__name__ != "dot_product" else 1000)
df = DataFrame(list(measure_time_dim("dot(va, vb)", ctxs, verbose=1)))
df["fct"] = fct.__name__
dfs.append(df)
print(df.tail(n=3))
0%| | 0/10 [00:00<?, ?it/s]
60%|██████ | 6/10 [00:00<00:00, 40.12it/s]
100%|██████████| 10/10 [00:00<00:00, 23.06it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
7 0.000123 0.000006 0.000118 0.000138 10 50 0.001227 184 0.000130 710 dot_product
8 0.000136 0.000002 0.000135 0.000140 10 50 0.001364 184 0.000146 810 dot_product
9 0.000179 0.000039 0.000153 0.000283 10 50 0.001787 184 0.000167 910 dot_product
0%| | 0/100 [00:00<?, ?it/s]
61%|██████ | 61/100 [00:00<00:00, 605.53it/s]
100%|██████████| 100/100 [00:00<00:00, 382.40it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000010 8.729575e-07 0.000008 0.000011 10 50 0.000095 184 0.000017 9710 ddot_cython_array
98 0.000013 1.537107e-06 0.000010 0.000016 10 50 0.000133 184 0.000019 9810 ddot_cython_array
99 0.000013 9.683444e-07 0.000011 0.000015 10 50 0.000126 184 0.000019 9910 ddot_cython_array
0%| | 0/100 [00:00<?, ?it/s]
67%|██████▋ | 67/100 [00:00<00:00, 647.22it/s]
100%|██████████| 100/100 [00:00<00:00, 464.35it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000007 1.895534e-07 0.000007 0.000007 10 50 0.000069 184 0.000013 9710 ddot_cython_array_optim
98 0.000007 9.850135e-08 0.000007 0.000007 10 50 0.000069 184 0.000012 9810 ddot_cython_array_optim
99 0.000007 1.462113e-07 0.000007 0.000007 10 50 0.000070 184 0.000012 9910 ddot_cython_array_optim
0%| | 0/100 [00:00<?, ?it/s]
66%|██████▌ | 66/100 [00:00<00:00, 646.16it/s]
100%|██████████| 100/100 [00:00<00:00, 454.00it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000007 1.668467e-07 0.000007 0.000007 10 50 0.000069 184 0.000013 9710 ddot_array
98 0.000007 2.074146e-07 0.000007 0.000008 10 50 0.000070 184 0.000012 9810 ddot_array
99 0.000007 1.312301e-07 0.000007 0.000007 10 50 0.000070 184 0.000014 9910 ddot_array
0%| | 0/100 [00:00<?, ?it/s]
93%|█████████▎| 93/100 [00:00<00:00, 915.30it/s]
100%|██████████| 100/100 [00:00<00:00, 865.69it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000004 2.483395e-07 0.000004 0.000004 10 50 0.000037 184 0.000012 9710 ddot_array_16
98 0.000004 9.604787e-08 0.000004 0.000004 10 50 0.000037 184 0.000012 9810 ddot_array_16
99 0.000004 1.166749e-07 0.000004 0.000004 10 50 0.000037 184 0.000012 9910 ddot_array_16
0%| | 0/100 [00:00<?, ?it/s]
99%|█████████▉| 99/100 [00:00<00:00, 977.99it/s]
100%|██████████| 100/100 [00:00<00:00, 970.38it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000003 9.790456e-08 0.000003 0.000003 10 50 0.000027 184 0.000012 9710 ddot_array_16_sse
98 0.000003 2.841234e-07 0.000003 0.000004 10 50 0.000029 184 0.000011 9810 ddot_array_16_sse
99 0.000003 1.359109e-08 0.000003 0.000003 10 50 0.000027 184 0.000015 9910 ddot_array_16_sse
Let’s display the results¶
cc = concat(dfs)
cc["N"] = cc["x_name"]
fig, ax = plt.subplots(2, 2, figsize=(10, 10))
cc[cc.N <= 1100].pivot(index="N", columns="fct", values="average").plot(
logy=True, logx=True, ax=ax[0, 0]
)
cc[cc.fct != "dot_product"].pivot(index="N", columns="fct", values="average").plot(
logy=True, ax=ax[0, 1]
)
cc[cc.fct != "dot_product"].pivot(index="N", columns="fct", values="average").plot(
logy=True, logx=True, ax=ax[1, 1]
)
ax[0, 0].set_title("Comparison of cython ddot implementations")
ax[0, 1].set_title("Comparison of cython ddot implementations\nwithout dot_product")
###################
# :epkg:`numpy` is faster but we are able to catch up.
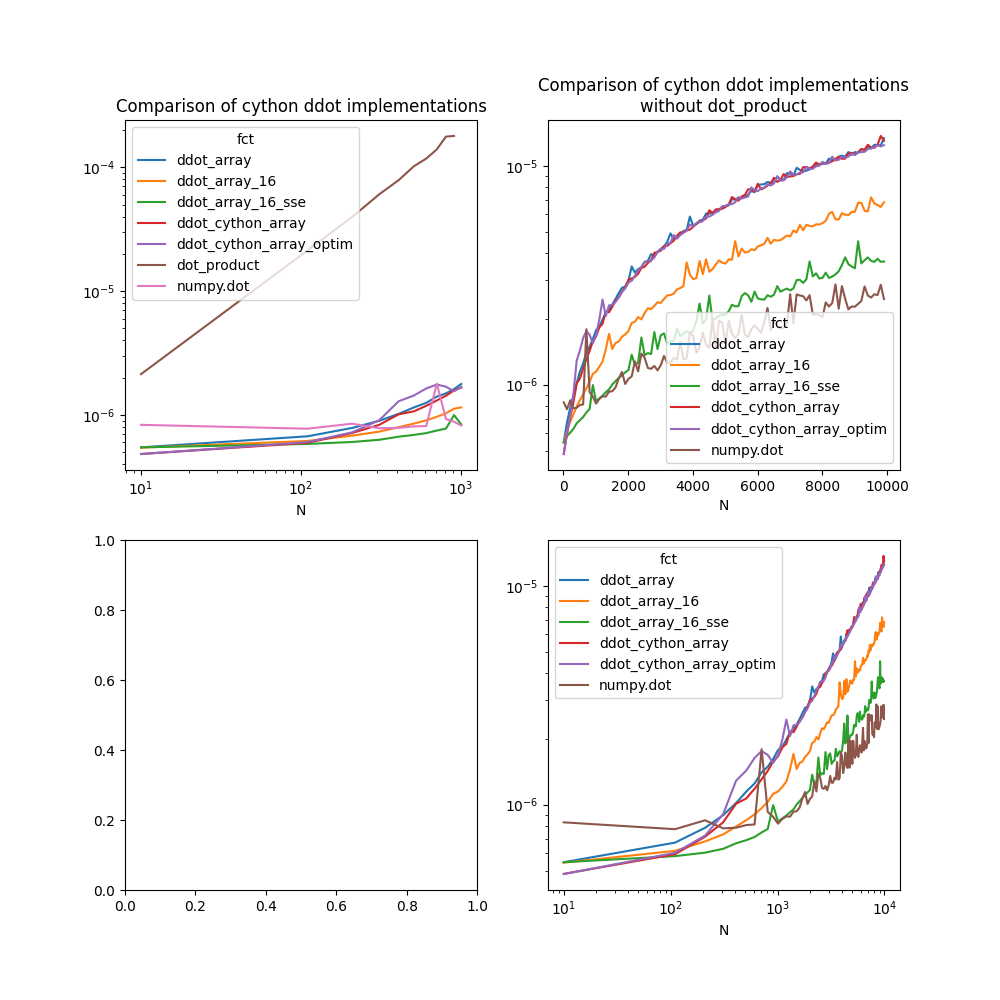
Text(0.5, 1.0, 'Comparison of cython ddot implementations\nwithout dot_product')
Same for floats¶
Let’s for single floats.
dfs = []
for fct in [
numpy.dot,
sdot_cython_array,
sdot_cython_array_optim,
sdot_array,
sdot_array_16,
sdot_array_16_sse,
]:
ctxs = get_vectors(
fct, 10000 if fct.__name__ != "dot_product" else 1000, dtype=numpy.float32
)
df = DataFrame(list(measure_time_dim("dot(va, vb)", ctxs, verbose=1)))
df["fct"] = fct.__name__
dfs.append(df)
print(df.tail(n=3))
cc = concat(dfs)
cc["N"] = cc["x_name"]
fig, ax = plt.subplots(1, 2, figsize=(10, 4))
cc.pivot(index="N", columns="fct", values="average").plot(logy=True, ax=ax[0])
cc.pivot(index="N", columns="fct", values="average").plot(
logy=True, logx=True, ax=ax[1]
)
ax[0].set_title("Comparison of cython sdot implementations")
ax[1].set_title("Comparison of cython sdot implementations")
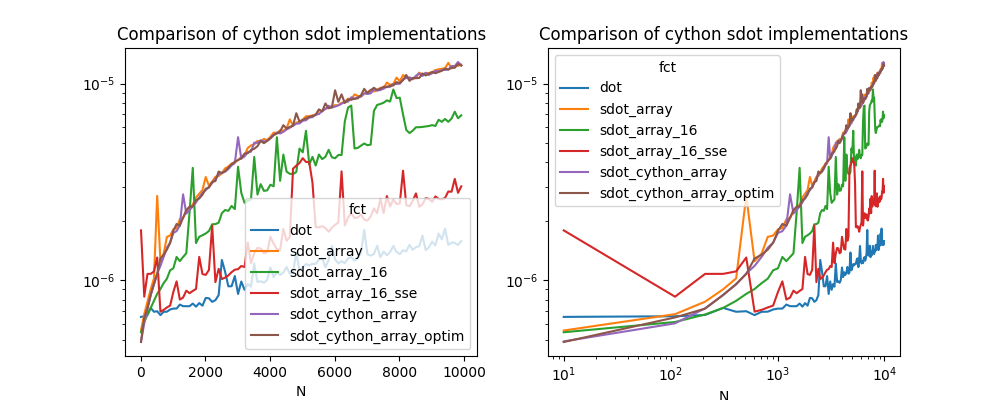
0%| | 0/100 [00:00<?, ?it/s]
100%|██████████| 100/100 [00:00<00:00, 1405.05it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000002 1.978880e-07 0.000002 0.000003 10 50 0.000025 184 0.000009 9710 dot
98 0.000003 1.251694e-07 0.000002 0.000003 10 50 0.000026 184 0.000008 9810 dot
99 0.000003 3.443201e-07 0.000003 0.000004 10 50 0.000030 184 0.000009 9910 dot
0%| | 0/100 [00:00<?, ?it/s]
69%|██████▉ | 69/100 [00:00<00:00, 681.52it/s]
100%|██████████| 100/100 [00:00<00:00, 480.58it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000007 1.068626e-07 0.000007 0.000007 10 50 0.000068 184 0.000012 9710 sdot_cython_array
98 0.000008 6.436713e-07 0.000007 0.000009 10 50 0.000085 184 0.000011 9810 sdot_cython_array
99 0.000010 3.937788e-07 0.000010 0.000011 10 50 0.000100 184 0.000015 9910 sdot_cython_array
0%| | 0/100 [00:00<?, ?it/s]
69%|██████▉ | 69/100 [00:00<00:00, 681.22it/s]
100%|██████████| 100/100 [00:00<00:00, 503.40it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000007 4.399225e-07 0.000007 0.000008 10 50 0.000069 184 0.000009 9710 sdot_cython_array_optim
98 0.000007 1.615574e-07 0.000007 0.000007 10 50 0.000069 184 0.000013 9810 sdot_cython_array_optim
99 0.000007 1.686930e-07 0.000007 0.000007 10 50 0.000070 184 0.000012 9910 sdot_cython_array_optim
0%| | 0/100 [00:00<?, ?it/s]
64%|██████▍ | 64/100 [00:00<00:00, 639.67it/s]
100%|██████████| 100/100 [00:00<00:00, 465.36it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000007 2.734671e-07 0.000007 0.000008 10 50 0.000069 184 0.000011 9710 sdot_array
98 0.000007 9.951844e-07 0.000007 0.000010 10 50 0.000074 184 0.000011 9810 sdot_array
99 0.000007 2.961736e-07 0.000007 0.000008 10 50 0.000073 184 0.000014 9910 sdot_array
0%| | 0/100 [00:00<?, ?it/s]
75%|███████▌ | 75/100 [00:00<00:00, 744.79it/s]
100%|██████████| 100/100 [00:00<00:00, 649.83it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000003 1.174517e-07 0.000003 0.000004 10 50 0.000033 184 0.000008 9710 sdot_array_16
98 0.000003 1.211944e-07 0.000003 0.000004 10 50 0.000034 184 0.000009 9810 sdot_array_16
99 0.000003 2.285007e-07 0.000003 0.000004 10 50 0.000034 184 0.000009 9910 sdot_array_16
0%| | 0/100 [00:00<?, ?it/s]
100%|██████████| 100/100 [00:00<00:00, 1283.17it/s]
average deviation min_exec max_exec repeat number ttime context_size warmup_time x_name fct
97 0.000002 6.594129e-07 0.000002 0.000004 10 50 0.000023 184 0.000008 9710 sdot_array_16_sse
98 0.000002 1.493392e-08 0.000002 0.000002 10 50 0.000021 184 0.000009 9810 sdot_array_16_sse
99 0.000002 1.304799e-07 0.000002 0.000003 10 50 0.000021 184 0.000007 9910 sdot_array_16_sse
Text(0.5, 1.0, 'Comparison of cython sdot implementations')
Total running time of the script: (0 minutes 3.620 seconds)