Note
Go to the end to download the full example code
Compares dot implementations (numpy, python, blas)¶
numpy has a very fast implementation of the dot product. It is difficult to be better and very easy to be slower. This example looks into a couple of slower implementations.
Compared implementations:
python dot: pydot¶
The first function pydot
uses
python to implement the dot product.
ctxs = [
dict(
va=numpy.random.randn(n).astype(numpy.float64),
vb=numpy.random.randn(n).astype(numpy.float64),
pydot=pydot,
x_name=n,
)
for n in range(10, 1000, 100)
]
res_pydot = list(measure_time_dim("pydot(va, vb)", ctxs, verbose=1))
pprint.pprint(res_pydot[:2])
0%| | 0/10 [00:00<?, ?it/s]
50%|█████ | 5/10 [00:00<00:00, 37.18it/s]
90%|█████████ | 9/10 [00:00<00:00, 23.38it/s]
100%|██████████| 10/10 [00:00<00:00, 22.38it/s]
[{'average': 7.600400000228547e-06,
'context_size': 232,
'deviation': 4.248885029711499e-07,
'max_exec': 8.723999999347142e-06,
'min_exec': 7.301999994524522e-06,
'number': 50,
'repeat': 10,
'ttime': 7.600400000228547e-05,
'warmup_time': 2.7599999611993553e-05,
'x_name': 10},
{'average': 5.5687400000351774e-05,
'context_size': 232,
'deviation': 5.511544605913856e-07,
'max_exec': 5.6999999997060514e-05,
'min_exec': 5.5131999997684036e-05,
'number': 50,
'repeat': 10,
'ttime': 0.0005568740000035178,
'warmup_time': 6.38999999864609e-05,
'x_name': 110}]
numpy dot¶
ctxs = [
dict(
va=numpy.random.randn(n).astype(numpy.float64),
vb=numpy.random.randn(n).astype(numpy.float64),
dot=numpy.dot,
x_name=n,
)
for n in range(10, 50000, 100)
]
res_dot = list(measure_time_dim("dot(va, vb)", ctxs, verbose=1))
pprint.pprint(res_dot[:2])
0%| | 0/500 [00:00<?, ?it/s]
21%|██ | 104/500 [00:00<00:00, 1019.90it/s]
41%|████ | 206/500 [00:09<00:15, 19.14it/s]
50%|████▉ | 249/500 [00:09<00:10, 22.97it/s]
55%|█████▌ | 275/500 [00:10<00:08, 27.48it/s]
60%|██████ | 300/500 [00:10<00:06, 33.32it/s]
66%|██████▌ | 329/500 [00:10<00:04, 42.73it/s]
71%|███████ | 355/500 [00:10<00:02, 53.55it/s]
76%|███████▌ | 381/500 [00:10<00:01, 67.23it/s]
81%|████████▏ | 407/500 [00:10<00:01, 81.63it/s]
86%|████████▌ | 431/500 [00:10<00:00, 98.48it/s]
91%|█████████ | 455/500 [00:10<00:00, 111.85it/s]
96%|█████████▌| 480/500 [00:10<00:00, 132.77it/s]
100%|██████████| 500/500 [00:11<00:00, 45.18it/s]
[{'average': 5.60800000130257e-07,
'context_size': 232,
'deviation': 4.489987402473388e-09,
'max_exec': 5.739999960496789e-07,
'min_exec': 5.580000015470432e-07,
'number': 50,
'repeat': 10,
'ttime': 5.60800000130257e-06,
'warmup_time': 1.249999968422344e-05,
'x_name': 10},
{'average': 5.733999987569405e-07,
'context_size': 232,
'deviation': 4.565084740193603e-09,
'max_exec': 5.839999994350365e-07,
'min_exec': 5.680000049324008e-07,
'number': 50,
'repeat': 10,
'ttime': 5.733999987569405e-06,
'warmup_time': 4.999999873689376e-06,
'x_name': 110}]
blas dot¶
numpy implementation uses BLAS. Let’s make a direct call to it.
0%| | 0/500 [00:00<?, ?it/s]
9%|▊ | 43/500 [00:00<00:01, 423.48it/s]
20%|█▉ | 99/500 [00:00<00:00, 501.04it/s]
30%|███ | 150/500 [00:00<00:00, 374.47it/s]
38%|███▊ | 191/500 [00:00<00:00, 334.48it/s]
45%|████▌ | 227/500 [00:00<00:00, 311.30it/s]
52%|█████▏ | 260/500 [00:00<00:00, 287.30it/s]
58%|█████▊ | 290/500 [00:00<00:00, 275.84it/s]
64%|██████▍ | 319/500 [00:01<00:00, 264.26it/s]
69%|██████▉ | 346/500 [00:01<00:00, 204.51it/s]
74%|███████▍ | 369/500 [00:01<00:00, 208.93it/s]
78%|███████▊ | 392/500 [00:01<00:00, 213.78it/s]
83%|████████▎ | 415/500 [00:01<00:00, 162.26it/s]
87%|████████▋ | 434/500 [00:02<00:00, 108.72it/s]
90%|████████▉ | 449/500 [00:05<00:02, 19.92it/s]
92%|█████████▏| 460/500 [00:07<00:03, 12.16it/s]
94%|█████████▎| 468/500 [00:08<00:02, 12.36it/s]
98%|█████████▊| 489/500 [00:08<00:00, 19.07it/s]
100%|█████████▉| 499/500 [00:08<00:00, 22.31it/s]
100%|██████████| 500/500 [00:08<00:00, 59.60it/s]
[{'average': 2.6987999990524263e-06,
'context_size': 360,
'deviation': 1.7646801380969342e-07,
'max_exec': 3.189999997630366e-06,
'min_exec': 2.598000000944012e-06,
'number': 50,
'repeat': 10,
'ttime': 2.6987999990524263e-05,
'warmup_time': 0.003485299999738345,
'x_name': 10},
{'average': 3.9423999987775465e-06,
'context_size': 360,
'deviation': 3.295376253108397e-06,
'max_exec': 1.3802000003124704e-05,
'min_exec': 2.7239999963057925e-06,
'number': 50,
'repeat': 10,
'ttime': 3.9423999987775465e-05,
'warmup_time': 1.2200000128359534e-05,
'x_name': 110}]
Let’s display the results¶
df1 = DataFrame(res_pydot)
df1["fct"] = "pydot"
df2 = DataFrame(res_dot)
df2["fct"] = "numpy.dot"
df3 = DataFrame(res_ddot)
df3["fct"] = "ddot"
cc = concat([df1, df2, df3])
cc["N"] = cc["x_name"]
fig, ax = plt.subplots(1, 2, figsize=(10, 4))
cc[cc.N <= 1100].pivot(index="N", columns="fct", values="average").plot(
logy=True, logx=True, ax=ax[0]
)
cc[cc.fct != "pydot"].pivot(index="N", columns="fct", values="average").plot(
logy=True, logx=True, ax=ax[1]
)
ax[0].set_title("Comparison of dot implementations")
ax[1].set_title("Comparison of dot implementations\nwithout python")
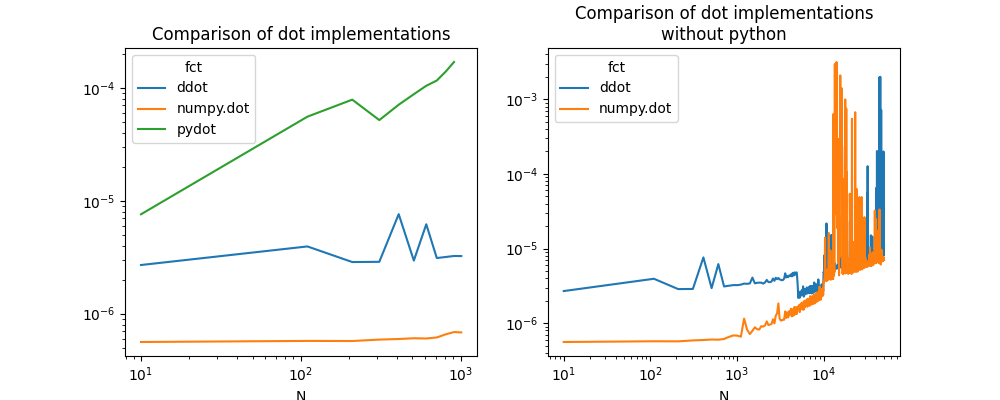
Text(0.5, 1.0, 'Comparison of dot implementations\nwithout python')
The results depends on the machine, its number of cores, the compilation settings of numpy or this module.
Total running time of the script: (0 minutes 21.163 seconds)