Note
Go to the end to download the full example code.
Do not use python int with dynamic shapes¶
torch.export.export()
uses torch.SymInt
to operate on shapes and
optimizes the graph it produces. It checks if two tensors share the same dimension,
if the shapes can be broadcast, … To do that, python types must not be used
or the algorithm looses information.
Wrong Model¶
import math
import torch
from onnx_diagnostic import doc
from onnx_diagnostic.torch_export_patches import torch_export_patches
class Model(torch.nn.Module):
def dim(self, i, divisor):
return int(math.ceil(i / divisor)) # noqa: RUF046
def forward(self, x):
new_shape = (self.dim(x.shape[0], 8), x.shape[1])
return torch.zeros(new_shape)
model = Model()
x = torch.rand((10, 15))
y = model(x)
print(f"x.shape={x.shape}, y.shape={y.shape}")
x.shape=torch.Size([10, 15]), y.shape=torch.Size([2, 15])
Export¶
DYN = torch.export.Dim.DYNAMIC
ep = torch.export.export(model, (x,), dynamic_shapes=(({0: DYN, 1: DYN}),))
print(ep)
ExportedProgram:
class GraphModule(torch.nn.Module):
def forward(self, x: "f32[s77, s27]"):
#
sym_size_int_3: "Sym(s27)" = torch.ops.aten.sym_size.int(x, 1); x = None
# File: ~/github/onnx-diagnostic/_doc/recipes/plot_dynamic_shapes_python_int.py:28 in forward, code: return torch.zeros(new_shape)
zeros: "f32[2, s27]" = torch.ops.aten.zeros.default([2, sym_size_int_3], device = device(type='cpu'), pin_memory = False); sym_size_int_3 = None
return (zeros,)
Graph signature:
# inputs
x: USER_INPUT
# outputs
zeros: USER_OUTPUT
Range constraints: {s77: VR[2, int_oo], s27: VR[2, int_oo]}
The last dimension became static. We must not use int.
math.ceil()
should be avoided as well since it is a python operation.
The exporter may fail to detect it is operating on shapes.
Rewrite¶
class RewrittenModel(torch.nn.Module):
def dim(self, i, divisor):
return (i + divisor - 1) // divisor
def forward(self, x):
new_shape = (self.dim(x.shape[0], 8), x.shape[1])
return torch.zeros(new_shape)
rewritten_model = RewrittenModel()
y = rewritten_model(x)
print(f"x.shape={x.shape}, y.shape={y.shape}")
x.shape=torch.Size([10, 15]), y.shape=torch.Size([2, 15])
Export¶
ep = torch.export.export(rewritten_model, (x,), dynamic_shapes=({0: DYN, 1: DYN},))
print(ep)
ExportedProgram:
class GraphModule(torch.nn.Module):
def forward(self, x: "f32[s77, s27]"):
#
sym_size_int_2: "Sym(s77)" = torch.ops.aten.sym_size.int(x, 0)
sym_size_int_3: "Sym(s27)" = torch.ops.aten.sym_size.int(x, 1); x = None
# File: ~/github/onnx-diagnostic/_doc/recipes/plot_dynamic_shapes_python_int.py:58 in forward, code: new_shape = (self.dim(x.shape[0], 8), x.shape[1])
add: "Sym(s77 + 8)" = sym_size_int_2 + 8; sym_size_int_2 = None
sub: "Sym(s77 + 7)" = add - 1; add = None
floordiv: "Sym(((s77 + 7)//8))" = sub // 8; sub = None
# File: ~/github/onnx-diagnostic/_doc/recipes/plot_dynamic_shapes_python_int.py:59 in forward, code: return torch.zeros(new_shape)
zeros: "f32[((s77 + 7)//8), s27]" = torch.ops.aten.zeros.default([floordiv, sym_size_int_3], device = device(type='cpu'), pin_memory = False); floordiv = sym_size_int_3 = None
return (zeros,)
Graph signature:
# inputs
x: USER_INPUT
# outputs
zeros: USER_OUTPUT
Range constraints: {s77: VR[2, int_oo], s27: VR[2, int_oo]}
Find the error¶
Function onnx_diagnostic.torch_export_patches.torch_export_patches()
has a parameter stop_if_static
which patches torch to raise exception
when something like that is happening.
with torch_export_patches(stop_if_static=True):
ep = torch.export.export(model, (x,), dynamic_shapes=({0: DYN, 1: DYN},))
print(ep)
ExportedProgram:
class GraphModule(torch.nn.Module):
def forward(self, x: "f32[s77, s27]"):
#
sym_size_int_3: "Sym(s27)" = torch.ops.aten.sym_size.int(x, 1); x = None
# File: ~/github/onnx-diagnostic/_doc/recipes/plot_dynamic_shapes_python_int.py:28 in forward, code: return torch.zeros(new_shape)
zeros: "f32[2, s27]" = torch.ops.aten.zeros.default([2, sym_size_int_3], device = device(type='cpu'), pin_memory = False); sym_size_int_3 = None
return (zeros,)
Graph signature:
# inputs
x: USER_INPUT
# outputs
zeros: USER_OUTPUT
Range constraints: {s77: VR[2, int_oo], s27: VR[2, int_oo]}
doc.plot_legend("dynamic shapes\ndo not cast to\npython int", "dynamic shapes", "yellow")
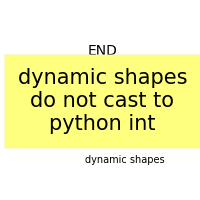
Related examples
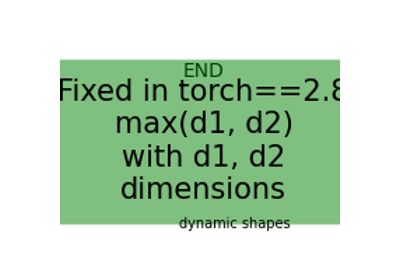
Cannot export torch.sym_max(x.shape[0], y.shape[0])
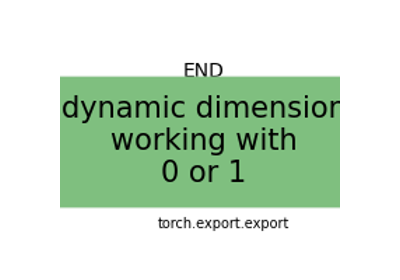
0, 1, 2 for a Dynamic Dimension in the dummy example to export a model