Note
Go to the end to download the full example code.
Intermediate results with (ONNX) ReferenceEvaluator¶
Let’s assume onnxruntime crashes without telling why or where. The first thing is do is to locate where. For that, we run a python runtime which is going to run until it fails.
A failing model¶
The issue here is a an operator Cast
trying to convert a result
into a non-existing type.
import numpy as np
import onnx
import onnx.helper as oh
import onnxruntime
from onnx_diagnostic import doc
from onnx_diagnostic.helpers.onnx_helper import from_array_extended
from onnx_diagnostic.reference import ExtendedReferenceEvaluator
TFLOAT = onnx.TensorProto.FLOAT
model = oh.make_model(
oh.make_graph(
[
oh.make_node("Mul", ["X", "Y"], ["xy"], name="n0"),
oh.make_node("Sigmoid", ["xy"], ["sy"], name="n1"),
oh.make_node("Add", ["sy", "one"], ["C"], name="n2"),
oh.make_node("Cast", ["C"], ["X999"], to=999, name="failing"),
oh.make_node("CastLike", ["X999", "Y"], ["Z"], name="n4"),
],
"-nd-",
[
oh.make_tensor_value_info("X", TFLOAT, ["a", "b", "c"]),
oh.make_tensor_value_info("Y", TFLOAT, ["a", "b", "c"]),
],
[oh.make_tensor_value_info("Z", TFLOAT, ["a", "b", "c"])],
[from_array_extended(np.array([1], dtype=np.float32), name="one")],
),
opset_imports=[oh.make_opsetid("", 18)],
ir_version=9,
)
We check it is failing.
try:
onnxruntime.InferenceSession(model.SerializeToString(), providers=["CPUExecutionProvider"])
except onnxruntime.capi.onnxruntime_pybind11_state.Fail as e:
print(e)
[ONNXRuntimeError] : 1 : FAIL : Node (failing) Op (Cast) [TypeInferenceError] Attribute to does not specify a valid type in .
ExtendedReferenceEvaluator¶
This class extends onnx.reference.ReferenceEvaluator
with operators outside the standard but defined by onnxruntime.
verbose=10 tells the class to print as much as possible,
verbose=0 prints nothing. Intermediate values for more or less verbosity.
ref = ExtendedReferenceEvaluator(model, verbose=10)
feeds = dict(
X=np.random.rand(3, 4).astype(np.float32), Y=np.random.rand(3, 4).astype(np.float32)
)
try:
ref.run(None, feeds)
except Exception as e:
print("ERROR", type(e), e)
+C one: float32:(1,):[1.0]
+I X: float32:(3, 4):0.2846825420856476,0.12602107226848602,0.4017603099346161,0.013444521464407444,0.026684239506721497...
+I Y: float32:(3, 4):0.051221176981925964,0.7418209910392761,0.025332288816571236,0.8432789444923401,0.34910184144973755...
Mul(X, Y) -> xy
+ xy: float32:(3, 4):0.014581775292754173,0.09348507970571518,0.010177507996559143,0.011337481439113617,0.00931551679968834...
Sigmoid(xy) -> sy
+ sy: float32:(3, 4):0.5036453604698181,0.5233542919158936,0.5025443434715271,0.5028343200683594,0.5023288726806641...
Add(sy, one) -> C
+ C: float32:(3, 4):1.503645420074463,1.5233542919158936,1.5025444030761719,1.5028343200683594,1.502328872680664...
Cast(C) -> X999
ERROR <class 'KeyError'> 999
We can see it run until it reaches Cast and stops. The error message is not always obvious to interpret. It gets improved every time from time to time. This runtime is useful when it fails for a numerical reason. It is possible to insert prints in the python code to print more information or debug if needed.
doc.plot_legend("Python Runtime\nfor ONNX", "ExtendedReferenceEvalutor", "lightgrey")
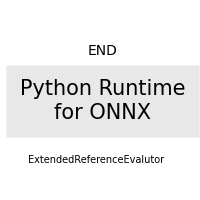
Total running time of the script: (0 minutes 0.056 seconds)
Related examples
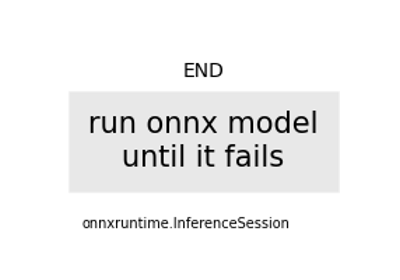
Find where a model is failing by running submodels
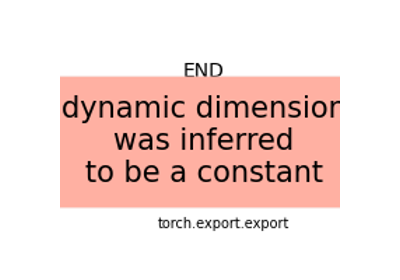
Find and fix an export issue due to dynamic shapes