Note
Go to the end to download the full example code.
First examples with onnxruntime¶
Example First examples with onnx-array-api defines a custom
loss and then executes it with class
onnx.reference.ReferenceEvaluator
.
Next example replaces it with onnxruntime.
Example¶
import numpy as np
from onnx_array_api.npx import absolute, jit_onnx
from onnx_array_api.ort.ort_tensors import JitOrtTensor, OrtTensor
def l1_loss(x, y):
return absolute(x - y).sum()
def l2_loss(x, y):
return ((x - y) ** 2).sum()
def myloss(x, y):
l1 = l1_loss(x[:, 0], y[:, 0])
l2 = l2_loss(x[:, 1], y[:, 1])
return l1 + l2
ort_myloss = jit_onnx(myloss, JitOrtTensor, target_opsets={"": 17}, ir_version=8)
x = np.array([[0.1, 0.2], [0.3, 0.4]], dtype=np.float32)
y = np.array([[0.11, 0.22], [0.33, 0.44]], dtype=np.float32)
xort = OrtTensor.from_array(x)
yort = OrtTensor.from_array(y)
res = ort_myloss(xort, yort)
print(res.numpy())
0.042
Profiling¶
from onnx_array_api.profiling import profile, profile2graph
x = np.random.randn(10000, 2).astype(np.float32)
y = np.random.randn(10000, 2).astype(np.float32)
xort = OrtTensor.from_array(x)
yort = OrtTensor.from_array(y)
def loop_ort(n):
for _ in range(n):
ort_myloss(xort, yort)
def loop_numpy(n):
for _ in range(n):
myloss(x, y)
def loop(n=1000):
loop_numpy(n)
loop_ort(n)
ps = profile(loop)[0]
root, nodes = profile2graph(ps, clean_text=lambda x: x.split("/")[-1])
text = root.to_text()
print(text)
isEnabledFor -- 8000 8000 -- 0.00371 0.00371 -- __init__.py:1724:isEnabledFor (isEnabledFor)
_leading_trailing -- 4000 20000 -- 0.06081 0.07644 -- arrayprint.py:398:_leading_trailing (_leading_trailing)
__getitem__ -- 16000 16000 -- 0.00818 0.01145 -- _index_tricks_impl.py:794:__getitem__ (__getitem__)
<built-in method builtins.isinstance> -- 16000 16000 -- 0.00327 0.00327 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
_leading_trailing -- 16000 8000 -- 0.02244 0.02930 -- arrayprint.py:398:_leading_trailing (_leading_trailing) +++
concatenate -- 4000 4000 -- 0.00139 0.00139 -- multiarray.py:161:concatenate (concatenate)
<built-in method builtins.len> -- 20000 20000 -- 0.00280 0.00280 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
recurser -- 4000 76000 -- 0.23592 0.55795 -- arrayprint.py:829:recurser (recurser)
_extendLine_pretty -- 48000 48000 -- 0.05146 0.13610 -- arrayprint.py:793:_extendLine_pretty (_extendLine_pretty)
_extendLine -- 48000 48000 -- 0.05162 0.06916 -- arrayprint.py:779:_extendLine (_extendLine)
<built-in method builtins.len> -- 192000 192000 -- 0.01753 0.01753 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<method 'splitlines' of 'str' objects> -- 48000 48000 -- 0.00925 0.00925 -- ~:0:<method 'splitlines' of 'str' objects> (<method 'splitlines' of 'str' objects>)
<built-in method builtins.len> -- 48000 48000 -- 0.00623 0.00623 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
recurser -- 72000 24000 -- 0.19099 0.51060 -- arrayprint.py:829:recurser (recurser) +++
__call__ -- 48000 48000 -- 0.09923 0.15462 -- arrayprint.py:1065:__call__ (__call__)
<built-in method numpy._c...math.dragon4_positional> -- 48000 48000 -- 0.05539 0.05539 -- ~:0:<built-in method numpy._core._multiarray_umath.dragon4_positional> (<built-in method numpy._core._multiarray_umath.dragon4_positional>) +++
<method 'rstrip' of 'str' objects> -- 28000 28000 -- 0.00481 0.00481 -- ~:0:<method 'rstrip' of 'str' objects> (<method 'rstrip' of 'str' objects>)
<built-in method builtins.len> -- 180000 180000 -- 0.01880 0.01880 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<built-in method builtins.max> -- 24000 24000 -- 0.00769 0.00769 -- ~:0:<built-in method builtins.max> (<built-in method builtins.max>) +++
_wrapreduction -- 8000 8000 -- 0.01772 0.05818 -- fromnumeric.py:69:_wrapreduction (_wrapreduction)
<dictcomp> -- 8000 8000 -- 0.00587 0.00587 -- fromnumeric.py:70:<dictcomp> (<dictcomp>)
<method 'items' of 'dict' objects> -- 8000 8000 -- 0.00153 0.00153 -- ~:0:<method 'items' of 'dict' objects> (<method 'items' of 'dict' objects>) +++
<method 'reduce' of 'numpy.ufunc' objects> -- 8000 8000 -- 0.03307 0.03307 -- ~:0:<method 'reduce' of 'numpy.ufunc' objects> (<method 'reduce' of 'numpy.ufunc' objects>) +++
var -- 2000 2000 -- 0.00250 0.02229 -- npx_core_api.py:54:var (var)
__init__ -- 2000 2000 -- 0.01426 0.01978 -- npx_var.py:287:__init__ (__init__) +++
info -- 5000 5000 -- 0.03017 1.45736 -- npx_jit_eager.py:52:info (info)
debug -- 4000 4000 -- 0.00301 0.00505 -- __init__.py:1455:debug (debug)
isEnabledFor -- 4000 4000 -- 0.00203 0.00203 -- __init__.py:1724:isEnabledFor (isEnabledFor) +++
info -- 4000 4000 -- 0.00244 0.00411 -- __init__.py:1467:info (info)
isEnabledFor -- 4000 4000 -- 0.00167 0.00167 -- __init__.py:1724:isEnabledFor (isEnabledFor) +++
__repr__ -- 4000 4000 -- 0.01911 1.41651 -- ort_tensors.py:165:__repr__ (__repr__)
_array_repr_implementation -- 4000 4000 -- 0.03160 1.38529 -- arrayprint.py:1561:_array_repr_implementation (_array_repr_implementation)
array2string -- 4000 4000 -- 0.02033 1.25291 -- arrayprint.py:595:array2string (array2string)
_make_options_dict -- 4000 4000 -- 0.01946 0.03471 -- arrayprint.py:50:_make_options_dict (_make_options_dict)
<dictcomp> -- 4000 4000 -- 0.00670 0.00670 -- arrayprint.py:59:<dictcomp> (<dictcomp>)
<method 'items' of 'dict' objects> -- 4000 4000 -- 0.00110 0.00110 -- ~:0:<method 'items' of 'dict' objects> (<method 'items' of 'dict' objects>) +++
<built-in method builtins.locals> -- 4000 4000 -- 0.00745 0.00745 -- ~:0:<built-in method builtins.locals> (<built-in method builtins.locals>)
wrapper -- 4000 4000 -- 0.01575 1.19308 -- arrayprint.py:540:wrapper (wrapper)
_array2string -- 4000 4000 -- 0.03199 1.17140 -- arrayprint.py:557:_array2string (_array2string)
_leading_trailing -- 4000 4000 -- 0.03837 0.07644 -- arrayprint.py:398:_leading_trailing (_leading_trailing) +++
_get_format_function -- 4000 4000 -- 0.02254 0.49635 -- arrayprint.py:486:_get_format_function (_get_format_function)
_get_formatdict -- 4000 4000 -- 0.01089 0.01089 -- arrayprint.py:436:_get_formatdict (_get_formatdict)
<lambda> -- 4000 4000 -- 0.00700 0.46043 -- arrayprint.py:445:<lambda> (<lambda>)
__init__ -- 4000 4000 -- 0.01579 0.45343 -- arrayprint.py:953:__init__ (__init__)
_none_or_positive_arg -- 4000 4000 -- 0.00166 0.00166 -- arrayprint.py:944:_none_or_positive_arg (_none_or_positive_arg)
fillFormat -- 4000 4000 -- 0.13107 0.43510 -- arrayprint.py:979:fillFormat (fillFormat)
__init__ -- 4000 4000 -- 0.00449 0.00449 -- _ufunc_config.py:431:__init__ (__init__)
__enter__ -- 4000 4000 -- 0.00728 0.01510 -- _ufunc_config.py:441:__enter__ (__enter__)
<method...jects> -- 4000 4000 -- 0.00384 0.00384 -- ~:0:<method 'set' of '_contextvars.ContextVar' objects> (<method 'set' of '_contextvars.ContextVar' objects>)
<built-...xtobj> -- 4000 4000 -- 0.00398 0.00398 -- ~:0:<built-in method numpy._core._multiarray_umath._make_extobj> (<built-in method numpy._core._multiarray_umath._make_extobj>)
__exit__ -- 4000 4000 -- 0.00313 0.00664 -- _ufunc_config.py:457:__exit__ (__exit__)
<method...jects> -- 4000 4000 -- 0.00352 0.00352 -- ~:0:<method 'reset' of '_contextvars.ContextVar' objects> (<method 'reset' of '_contextvars.ContextVar' objects>)
<genexpr> -- 52000 52000 -- 0.03463 0.14049 -- arrayprint.py:1034:<genexpr> (<genexpr>)
<genexpr> -- 52000 52000 -- 0.04079 0.09334 -- arrayprint.py:1029:<genexpr> (<genexpr>)
<buil...nal> -- 48000 48000 -- 0.05255 0.05255 -- ~:0:<built-in method numpy._core._multiarray_umath.dragon4_positional> (<built-in method numpy._core._multiarray_umath.dragon4_positional>) +++
<method...jects> -- 48000 48000 -- 0.01252 0.01252 -- ~:0:<method 'split' of 'str' objects> (<method 'split' of 'str' objects>)
_max_dispatcher -- 4000 4000 -- 0.00135 0.00135 -- fromnumeric.py:3075:_max_dispatcher (_max_dispatcher)
max -- 4000 4000 -- 0.00766 0.04619 -- fromnumeric.py:3080:max (max)
_wrapreduction -- 4000 4000 -- 0.01088 0.03853 -- fromnumeric.py:69:_wrapreduction (_wrapreduction) +++
_min_dispatcher -- 4000 4000 -- 0.00104 0.00104 -- fromnumeric.py:3220:_min_dispatcher (_min_dispatcher)
min -- 4000 4000 -- 0.00498 0.02464 -- fromnumeric.py:3225:min (min)
_wrapreduction -- 4000 4000 -- 0.00685 0.01966 -- fromnumeric.py:69:_wrapreduction (_wrapreduction) +++
<built-in...ins.len> -- 8000 8000 -- 0.00173 0.00173 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<built-in...ins.max> -- 8000 8000 -- 0.02270 0.06235 -- ~:0:<built-in method builtins.max> (<built-in method builtins.max>) +++
<built-in m...sinstance> -- 4000 4000 -- 0.00087 0.00087 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in metho...ns.issubclass> -- 16000 16000 -- 0.00249 0.00249 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>) +++
_formatArray -- 4000 4000 -- 0.00693 0.56488 -- arrayprint.py:820:_formatArray (_formatArray)
recurser -- 4000 4000 -- 0.04493 0.55795 -- arrayprint.py:829:recurser (recurser) +++
<built-in method builtins.len> -- 4000 4000 -- 0.00044 0.00044 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<built-in method numpy.asarray> -- 4000 4000 -- 0.00130 0.00130 -- ~:0:<built-in method numpy.asarray> (<built-in method numpy.asarray>)
<method 'add' of 'set' objects> -- 4000 4000 -- 0.00141 0.00141 -- ~:0:<method 'add' of 'set' objects> (<method 'add' of 'set' objects>)
<method 'discard' of 'set' objects> -- 4000 4000 -- 0.00180 0.00180 -- ~:0:<method 'discard' of 'set' objects> (<method 'discard' of 'set' objects>)
<built-in method builtins.id> -- 4000 4000 -- 0.00146 0.00146 -- ~:0:<built-in method builtins.id> (<built-in method builtins.id>)
<built-in method _thread.get_ident> -- 4000 4000 -- 0.00125 0.00125 -- ~:0:<built-in method _thread.get_ident> (<built-in method _thread.get_ident>)
<method 'get' of '_co...ContextVar' objects> -- 4000 4000 -- 0.00071 0.00071 -- ~:0:<method 'get' of '_contextvars.ContextVar' objects> (<method 'get' of '_contextvars.ContextVar' objects>) +++
<method 'update' of 'dict' objects> -- 4000 4000 -- 0.00180 0.00180 -- ~:0:<method 'update' of 'dict' objects> (<method 'update' of 'dict' objects>)
<method 'copy' of 'dict' objects> -- 4000 4000 -- 0.00165 0.00165 -- ~:0:<method 'copy' of 'dict' objects> (<method 'copy' of 'dict' objects>)
<built-in method builtins.len> -- 4000 4000 -- 0.00064 0.00064 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
dtype_is_implied -- 4000 4000 -- 0.00964 0.01032 -- arrayprint.py:1487:dtype_is_implied (dtype_is_implied)
<method 'get' of '_co...ContextVar' objects> -- 4000 4000 -- 0.00068 0.00068 -- ~:0:<method 'get' of '_contextvars.ContextVar' objects> (<method 'get' of '_contextvars.ContextVar' objects>) +++
dtype_short_repr -- 4000 4000 -- 0.02942 0.08308 -- arrayprint.py:1529:dtype_short_repr (dtype_short_repr)
_name_get -- 4000 4000 -- 0.01635 0.05022 -- _dtype.py:350:_name_get (_name_get)
_kind_name -- 4000 4000 -- 0.00311 0.00311 -- _dtype.py:24:_kind_name (_kind_name)
_name_includes_bit_suffix -- 4000 4000 -- 0.00681 0.02530 -- _dtype.py:334:_name_includes_bit_suffix (_name_includes_bit_suffix)
issubdtype -- 4000 4000 -- 0.00773 0.01849 -- numerictypes.py:471:issubdtype (issubdtype)
issubclass_ -- 8000 8000 -- 0.00725 0.01022 -- numerictypes.py:289:issubclass_ (issubclass_)
<built-in met....issubclass> -- 8000 8000 -- 0.00297 0.00297 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>) +++
<built-in metho...ns.issubclass> -- 4000 4000 -- 0.00054 0.00054 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>) +++
<method 'format' of 'str' objects> -- 4000 4000 -- 0.00483 0.00483 -- ~:0:<method 'format' of 'str' objects> (<method 'format' of 'str' objects>) +++
<built-in method builtins.issubclass> -- 4000 4000 -- 0.00063 0.00063 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>) +++
<method 'isalpha' of 'str' objects> -- 4000 4000 -- 0.00116 0.00116 -- ~:0:<method 'isalpha' of 'str' objects> (<method 'isalpha' of 'str' objects>)
<method 'isalnum' of 'str' objects> -- 4000 4000 -- 0.00134 0.00134 -- ~:0:<method 'isalnum' of 'str' objects> (<method 'isalnum' of 'str' objects>)
<built-in method builtins.issubclass> -- 4000 4000 -- 0.00094 0.00094 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>) +++
<method 'rfind' of 'str' objects> -- 4000 4000 -- 0.00184 0.00184 -- ~:0:<method 'rfind' of 'str' objects> (<method 'rfind' of 'str' objects>)
<method 'format' of 'str' objects> -- 4000 4000 -- 0.00293 0.00293 -- ~:0:<method 'format' of 'str' objects> (<method 'format' of 'str' objects>) +++
<method 'get' of '_cont...s.ContextVar' objects> -- 4000 4000 -- 0.00141 0.00141 -- ~:0:<method 'get' of '_contextvars.ContextVar' objects> (<method 'get' of '_contextvars.ContextVar' objects>) +++
<built-in method builtins.len> -- 8000 8000 -- 0.00120 0.00120 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
numpy -- 4000 4000 -- 0.01211 0.01211 -- ort_tensors.py:61:numpy (numpy)
<method 'get' of 'dict' objects> -- 2000 2000 -- 0.00065 0.00065 -- ~:0:<method 'get' of 'dict' objects> (<method 'get' of 'dict' objects>)
<built-in method builtins.len> -- 4000 4000 -- 0.00087 0.00087 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
get_cst_var -- 2000 2000 -- 0.00467 0.00656 -- npx_var.py:216:get_cst_var (get_cst_var)
parent -- 2000 2000 -- 0.00139 0.00189 -- <frozen importlib._bootstrap>:404:parent (parent)
<method 'rpartition' of 'str' objects> -- 2000 2000 -- 0.00050 0.00050 -- ~:0:<method 'rpartition' of 'str' objects> (<method 'rpartition' of 'str' objects>)
__init__ -- 3000 3000 -- 0.02318 0.03204 -- npx_var.py:287:__init__ (__init__)
__init__ -- 3000 3000 -- 0.00084 0.00084 -- npx_var.py:281:__init__ (__init__)
<listcomp> -- 3000 3000 -- 0.00090 0.00090 -- npx_var.py:345:<listcomp> (<listcomp>)
self_var -- 2000 2000 -- 0.00079 0.00106 -- npx_var.py:375:self_var (self_var) +++
<method 'items' of 'dict' objects> -- 3000 3000 -- 0.00042 0.00042 -- ~:0:<method 'items' of 'dict' objects> (<method 'items' of 'dict' objects>) +++
<built-in method builtins.hasattr> -- 3000 3000 -- 0.00047 0.00047 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.isinstance> -- 23000 23000 -- 0.00385 0.00385 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.len> -- 6000 6000 -- 0.00095 0.00095 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<method 'ravel' of 'numpy.ndarray' objects> -- 1000 1000 -- 0.00039 0.00039 -- ~:0:<method 'ravel' of 'numpy.ndarray' objects> (<method 'ravel' of 'numpy.ndarray' objects>)
self_var -- 4000 4000 -- 0.00171 0.00235 -- npx_var.py:375:self_var (self_var)
<built-in method builtins.hasattr> -- 4000 4000 -- 0.00064 0.00064 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
__init__ -- 3000 3000 -- 0.00461 0.00710 -- ort_tensors.py:144:__init__ (__init__)
<built-in method builtins.isinstance> -- 5000 5000 -- 0.00249 0.00249 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
value -- 3000 3000 -- 0.00094 0.00094 -- ort_tensors.py:192:value (value)
loop -- 1 1 -- 0.00000 2.50002 -- plot_onnxruntime.py:66:loop (loop)
loop_ort -- 1 1 -- 0.00580 2.26451 -- plot_onnxruntime.py:56:loop_ort (loop_ort)
__call__ -- 1000 1000 -- 0.00612 2.25871 -- npx_jit_eager.py:584:__call__ (__call__)
info -- 2000 2000 -- 0.00589 0.00811 -- npx_jit_eager.py:52:info (info) +++
cast_to_tensor_class -- 1000 1000 -- 0.00460 0.00938 -- npx_jit_eager.py:394:cast_to_tensor_class (cast_to_tensor_class)
__init__ -- 2000 2000 -- 0.00228 0.00426 -- ort_tensors.py:144:__init__ (__init__) +++
<method 'append' of 'list' objects> -- 2000 2000 -- 0.00052 0.00052 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
cast_from_tensor_class -- 1000 1000 -- 0.00273 0.00393 -- npx_jit_eager.py:411:cast_from_tensor_class (cast_from_tensor_class)
value -- 1000 1000 -- 0.00034 0.00034 -- ort_tensors.py:192:value (value) +++
<built-in method builtins.isinstance> -- 1000 1000 -- 0.00063 0.00063 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.len> -- 1000 1000 -- 0.00023 0.00023 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
jit_call -- 1000 1000 -- 0.01538 2.23115 -- npx_jit_eager.py:462:jit_call (jit_call)
info -- 3000 3000 -- 0.02428 1.44925 -- npx_jit_eager.py:52:info (info) +++
make_key -- 1000 1000 -- 0.00657 0.02072 -- npx_jit_eager.py:151:make_key (make_key)
key -- 2000 2000 -- 0.00285 0.01268 -- ort_tensors.py:187:key (key)
shape -- 2000 2000 -- 0.00240 0.00240 -- ort_tensors.py:177:shape (shape)
dtype -- 2000 2000 -- 0.00442 0.00708 -- ort_tensors.py:182:dtype (dtype)
__init__ -- 2000 2000 -- 0.00193 0.00266 -- npx_types.py:29:__init__ (__init__)
<built-in met....isinstance> -- 4000 4000 -- 0.00072 0.00072 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.len> -- 2000 2000 -- 0.00035 0.00035 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<method 'append' of 'list' objects> -- 2000 2000 -- 0.00043 0.00043 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
<built-in method builtins.isinstance> -- 2000 2000 -- 0.00104 0.00104 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
move_input_to_kwargs -- 1000 1000 -- 0.00084 0.00084 -- npx_jit_eager.py:426:move_input_to_kwargs (move_input_to_kwargs)
__hash__ -- 4000 4000 -- 0.00096 0.00096 -- npx_types.py:45:__hash__ (__hash__)
__eq__ -- 4000 4000 -- 0.00172 0.00172 -- npx_types.py:56:__eq__ (__eq__)
run -- 1000 1000 -- 0.73854 0.74230 -- ort_tensors.py:124:run (run)
__init__ -- 1000 1000 -- 0.00233 0.00283 -- ort_tensors.py:144:__init__ (__init__) +++
value -- 2000 2000 -- 0.00060 0.00060 -- ort_tensors.py:192:value (value) +++
<built-in method builtins.len> -- 2000 2000 -- 0.00033 0.00033 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
loop_numpy -- 1 1 -- 0.00061 0.23550 -- plot_onnxruntime.py:61:loop_numpy (loop_numpy)
myloss -- 1000 1000 -- 0.00301 0.23489 -- plot_onnxruntime.py:28:myloss (myloss)
__add__ -- 1000 1000 -- 0.00062 0.02115 -- npx_var.py:645:__add__ (__add__)
_binary_op -- 1000 1000 -- 0.00341 0.02053 -- npx_var.py:615:_binary_op (_binary_op)
var -- 1000 1000 -- 0.00145 0.01276 -- npx_core_api.py:54:var (var) +++
get_cst_var -- 1000 1000 -- 0.00233 0.00336 -- npx_var.py:216:get_cst_var (get_cst_var) +++
self_var -- 1000 1000 -- 0.00045 0.00064 -- npx_var.py:375:self_var (self_var) +++
<built-in method builtins.isinstance> -- 1000 1000 -- 0.00037 0.00037 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
l1_loss -- 1000 1000 -- 0.01209 0.18592 -- plot_onnxruntime.py:20:l1_loss (l1_loss)
wrapper -- 1000 1000 -- 0.14128 0.15795 -- npx_core_api.py:142:wrapper (wrapper)
annotation -- 1000 1000 -- 0.00017 0.00017 -- inspect.py:2695:annotation (annotation)
kind -- 2000 2000 -- 0.00033 0.00033 -- inspect.py:2699:kind (kind)
parameters -- 2000 2000 -- 0.00053 0.00053 -- inspect.py:3006:parameters (parameters)
return_annotation -- 1000 1000 -- 0.00017 0.00017 -- inspect.py:3010:return_annotation (return_annotation)
__init__ -- 1000 1000 -- 0.00891 0.01225 -- npx_var.py:287:__init__ (__init__) +++
<method 'items' of ...ingproxy' objects> -- 1000 1000 -- 0.00031 0.00031 -- ~:0:<method 'items' of 'mappingproxy' objects> (<method 'items' of 'mappingproxy' objects>)
<method 'items' of 'dict' objects> -- 1000 1000 -- 0.00015 0.00015 -- ~:0:<method 'items' of 'dict' objects> (<method 'items' of 'dict' objects>) +++
<method 'append' of 'list' objects> -- 1000 1000 -- 0.00020 0.00020 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
<built-in method builtins.any> -- 1000 1000 -- 0.00069 0.00170 -- ~:0:<built-in method builtins.any> (<built-in method builtins.any>)
<genexpr> -- 2000 2000 -- 0.00081 0.00101 -- npx_core_api.py:143:<genexpr> (<genexpr>)
<built-in metho...ns.isinstance> -- 1000 1000 -- 0.00020 0.00020 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.isinstance> -- 2000 2000 -- 0.00035 0.00035 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.issubclass> -- 2000 2000 -- 0.00035 0.00035 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>) +++
<built-in method builtins.len> -- 1000 1000 -- 0.00016 0.00016 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
sum -- 1000 1000 -- 0.00086 0.01588 -- npx_var.py:890:sum (sum)
reduce_function -- 1000 1000 -- 0.00164 0.01502 -- npx_var.py:871:reduce_function (reduce_function)
var -- 1000 1000 -- 0.00105 0.00953 -- npx_core_api.py:54:var (var) +++
get_cst_var -- 1000 1000 -- 0.00234 0.00320 -- npx_var.py:216:get_cst_var (get_cst_var) +++
self_var -- 1000 1000 -- 0.00047 0.00065 -- npx_var.py:375:self_var (self_var) +++
l2_loss -- 1000 1000 -- 0.01669 0.02480 -- plot_onnxruntime.py:24:l2_loss (l2_loss)
<method 'sum' of 'numpy.ndarray' objects> -- 1000 1000 -- 0.00060 0.00811 -- ~:0:<method 'sum' of 'numpy.ndarray' objects> (<method 'sum' of 'numpy.ndarray' objects>)
_sum -- 1000 1000 -- 0.00059 0.00750 -- _methods.py:51:_sum (_sum)
<method 'reduce' ....ufunc' objects> -- 1000 1000 -- 0.00691 0.00691 -- ~:0:<method 'reduce' of 'numpy.ufunc' objects> (<method 'reduce' of 'numpy.ufunc' objects>) +++
<built-in method builtins.len> -- 576000 576000 -- 0.06291 0.06291 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>)
<method 'append' of 'list' objects> -- 5000 5000 -- 0.00115 0.00115 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>)
<built-in method builtins.isinstance> -- 59000 59000 -- 0.01379 0.01379 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>)
<method 'items' of 'dict' objects> -- 16000 16000 -- 0.00319 0.00319 -- ~:0:<method 'items' of 'dict' objects> (<method 'items' of 'dict' objects>)
<built-in method builtins.issubclass> -- 38000 38000 -- 0.00793 0.00793 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>)
<method 'format' of 'str' objects> -- 8000 8000 -- 0.00776 0.00776 -- ~:0:<method 'format' of 'str' objects> (<method 'format' of 'str' objects>)
<method 'get' of '_contextvars.ContextVar' objects> -- 12000 12000 -- 0.00280 0.00280 -- ~:0:<method 'get' of '_contextvars.ContextVar' objects> (<method 'get' of '_contextvars.ContextVar' objects>)
<built-in method builtins.hasattr> -- 7000 7000 -- 0.00110 0.00110 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>)
<method 'reduce' of 'numpy.ufunc' objects> -- 9000 9000 -- 0.03998 0.03998 -- ~:0:<method 'reduce' of 'numpy.ufunc' objects> (<method 'reduce' of 'numpy.ufunc' objects>)
<built-in method builtins.max> -- 32000 32000 -- 0.03039 0.07004 -- ~:0:<built-in method builtins.max> (<built-in method builtins.max>)
<genexpr> -- 52000 52000 -- 0.01578 0.02168 -- arrayprint.py:1038:<genexpr> (<genexpr>)
<built-in method builtins.len> -- 48000 48000 -- 0.00590 0.00590 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<genexpr> -- 52000 52000 -- 0.01320 0.01798 -- arrayprint.py:1039:<genexpr> (<genexpr>)
<built-in method builtins.len> -- 48000 48000 -- 0.00477 0.00477 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
<built-in method numpy._core....ay_umath.dragon4_positional> -- 96000 96000 -- 0.10794 0.10794 -- ~:0:<built-in method numpy._core._multiarray_umath.dragon4_positional> (<built-in method numpy._core._multiarray_umath.dragon4_positional>)
Benchmark¶
from pandas import DataFrame
from tqdm import tqdm
from onnx_array_api.ext_test_case import measure_time
data = []
for n in tqdm([1, 10, 100, 1000, 10000, 100000]):
x = np.random.randn(n, 2).astype(np.float32)
y = np.random.randn(n, 2).astype(np.float32)
obs = measure_time(lambda x=x, y=y: myloss(x, y))
obs["name"] = "numpy"
obs["n"] = n
data.append(obs)
xort = OrtTensor.from_array(x)
yort = OrtTensor.from_array(y)
obs = measure_time(lambda xort=xort, yort=yort: ort_myloss(xort, yort))
obs["name"] = "ort"
obs["n"] = n
data.append(obs)
df = DataFrame(data)
piv = df.pivot(index="n", columns="name", values="average")
piv
0%| | 0/6 [00:00<?, ?it/s]
17%|█▋ | 1/6 [00:00<00:02, 1.86it/s]
33%|███▎ | 2/6 [00:01<00:02, 1.44it/s]
50%|█████ | 3/6 [00:04<00:05, 1.82s/it]
67%|██████▋ | 4/6 [00:05<00:02, 1.42s/it]
83%|████████▎ | 5/6 [00:06<00:01, 1.20s/it]
100%|██████████| 6/6 [00:07<00:00, 1.16s/it]
100%|██████████| 6/6 [00:07<00:00, 1.20s/it]
Plots¶
import matplotlib.pyplot as plt
fig, ax = plt.subplots(1, 2, figsize=(12, 4))
piv.plot(
title="Comparison between numpy and onnxruntime", logx=True, logy=True, ax=ax[0]
)
piv["ort/numpy"] = piv["ort"] / piv["numpy"]
piv["ort/numpy"].plot(title="Ratio ort/numpy", logx=True, ax=ax[1])
fig.savefig("plot_onnxruntime.png")
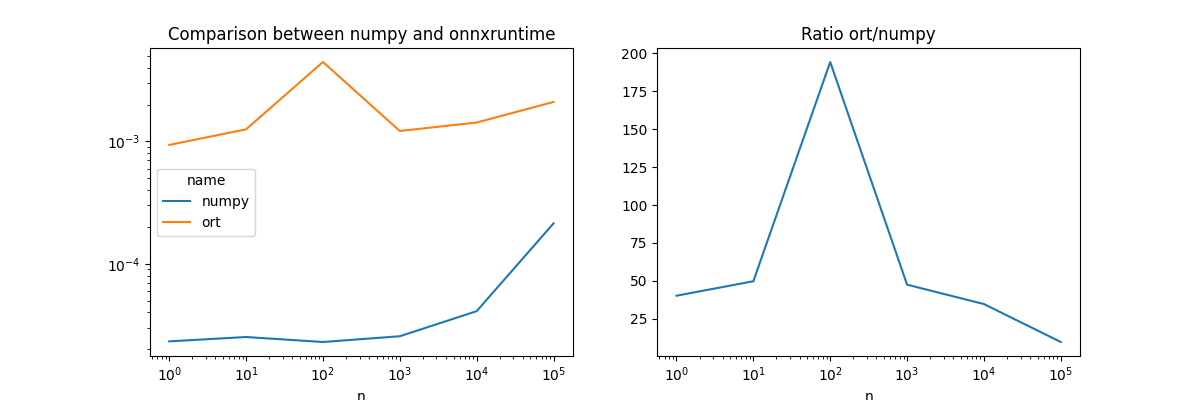
Total running time of the script: (0 minutes 17.941 seconds)