Note
Go to the end to download the full example code
Measuring performance about Gemm with onnxruntime#
The benchmark measures the performance of Gemm for different types and configuration. That includes a custom operator only available on CUDA calling function cublasLtMatmul. This function offers many options.
import pprint
import platform
from itertools import product
import numpy
from tqdm import tqdm
import matplotlib.pyplot as plt
from pandas import DataFrame, pivot_table
from onnx import TensorProto
from onnx.helper import (
make_model,
make_node,
make_graph,
make_tensor_value_info,
make_opsetid,
)
from onnx.checker import check_model
from onnx.numpy_helper import from_array
from onnx.reference import ReferenceEvaluator
from onnxruntime import InferenceSession, SessionOptions, get_available_providers
from onnxruntime.capi._pybind_state import (
OrtValue as C_OrtValue,
OrtDevice as C_OrtDevice,
)
from onnxruntime.capi.onnxruntime_pybind11_state import (
Fail,
NotImplemented,
InvalidGraph,
InvalidArgument,
)
try:
from onnx_array_api.plotting.text_plot import onnx_simple_text_plot
except ImportError:
onnx_simple_text_plot = str
try:
from onnx_extended.reference import CReferenceEvaluator
except ImportError:
CReferenceEvaluator = ReferenceEvaluator
from onnx_extended.ext_test_case import unit_test_going, measure_time, get_parsed_args
try:
from onnx_extended.validation.cuda.cuda_example_py import get_device_prop
from onnx_extended.ortops.tutorial.cuda import get_ort_ext_libs
has_cuda = True
except ImportError:
def get_device_prop():
return {"name": "CPU"}
def get_ort_ext_libs():
return None
has_cuda = False
default_dims = (
"32,32,32;64,64,64;128,128,128;256,256,256;"
"400,400,400;512,512,512;1024,1024,1024"
)
if has_cuda:
prop = get_device_prop()
if prop.get("major", 0) >= 7:
default_dims += ";2048,2048,2048;4096,4096,4096"
if prop.get("major", 0) >= 9:
default_dims += ";16384,16384,16384"
script_args = get_parsed_args(
"plot_bench_gemm_ort",
description=__doc__,
dims=(
"32,32,32;64,64,64" if unit_test_going() else default_dims,
"square matrix dimensions to try, comma separated values",
),
types=(
"FLOAT" if unit_test_going() else "FLOAT8E4M3FN,FLOAT,FLOAT16,BFLOAT16",
"element type to teest",
),
number=2 if unit_test_going() else 4,
repeat=2 if unit_test_going() else 10,
warmup=2 if unit_test_going() else 5,
expose="repeat,number,warmup",
)
Device properties#
if has_cuda:
properties = get_device_prop()
pprint.pprint(properties)
else:
properties = {"major": 0}
{'clockRate': 1569000,
'computeMode': 0,
'concurrentKernels': 1,
'isMultiGpuBoard': 0,
'major': 6,
'maxThreadsPerBlock': 1024,
'minor': 1,
'multiProcessorCount': 10,
'name': 'NVIDIA GeForce GTX 1060',
'sharedMemPerBlock': 49152,
'totalConstMem': 65536,
'totalGlobalMem': 6442319872}
Model to benchmark#
It includes one Gemm. The operator changes. It can the regular Gemm, a custom Gemm from domain com.microsoft or a custom implementation from domain onnx_extented.ortops.tutorial.cuda.
def create_model(
mat_type=TensorProto.FLOAT, provider="CUDAExecutionProvider", domain="com.microsoft"
):
A = make_tensor_value_info("A", mat_type, [None, None])
B = make_tensor_value_info("B", mat_type, [None, None])
outputs = [make_tensor_value_info("C", mat_type, [None, None])]
inits = []
if domain != "":
if provider != "CUDAExecutionProvider":
return None
f8 = False
if domain == "com.microsoft":
op_name = "GemmFloat8"
computeType = "CUBLAS_COMPUTE_32F"
node_output = ["C"]
elif mat_type == TensorProto.FLOAT:
op_name = "CustomGemmFloat"
computeType = "CUBLAS_COMPUTE_32F_FAST_TF32"
node_output = ["C"]
elif mat_type == TensorProto.FLOAT16:
op_name = "CustomGemmFloat16"
computeType = "CUBLAS_COMPUTE_16F"
node_output = ["C"]
elif mat_type in (TensorProto.FLOAT8E4M3FN, TensorProto.FLOAT8E5M2):
f8 = True
op_name = "CustomGemmFloat8E4M3FN"
computeType = "CUBLAS_COMPUTE_32F"
node_output = ["C"]
outputs = [
make_tensor_value_info("C", TensorProto.FLOAT16, [None, None]),
]
inits.append(from_array(numpy.array([1], dtype=numpy.float32), name="I"))
else:
return None
node_kw = dict(
alpha=1.0,
transB=1,
domain=domain,
computeType=computeType,
fastAccumulationMode=1,
rowMajor=0 if op_name.startswith("CustomGemmFloat") else 1,
)
node_kw["name"] = (
f"{mat_type}.{len(node_output)}.{len(outputs)}."
f"{domain}..{node_kw['rowMajor']}.."
f"{node_kw['fastAccumulationMode']}..{node_kw['computeType']}.."
f"{f8}"
)
node_inputs = ["A", "B"]
if f8:
node_inputs.append("")
node_inputs.extend(["I"] * 3)
nodes = [make_node(op_name, node_inputs, node_output, **node_kw)]
else:
nodes = [
make_node("Gemm", ["A", "B"], ["C"], transA=1, beta=0.0),
]
graph = make_graph(nodes, "a", [A, B], outputs, inits)
if mat_type < 16:
# regular type
opset, ir = 18, 8
else:
opset, ir = 19, 9
onnx_model = make_model(
graph,
opset_imports=[
make_opsetid("", opset),
make_opsetid("com.microsoft", 1),
make_opsetid("onnx_extented.ortops.tutorial.cuda", 1),
],
ir_version=ir,
)
check_model(onnx_model)
return onnx_model
print(onnx_simple_text_plot(create_model()))
opset: domain='' version=18
opset: domain='com.microsoft' version=1
opset: domain='onnx_extented.ortops.tutorial.cuda' version=1
input: name='A' type=dtype('float32') shape=['', '']
input: name='B' type=dtype('float32') shape=['', '']
GemmFloat8[com.microsoft](A, B, alpha=1.00, computeType=b'CUBLAS_COMPUTE_32F', fastAccumulationMode=1, rowMajor=1, transB=1) -> C
output: name='C' type=dtype('float32') shape=['', '']
A model to cast into anytype. numpy does not support float 8. onnxruntime is used to cast a float array into any type. It must be called with tensor of type OrtValue.
def create_cast(to, cuda=False):
A = make_tensor_value_info("A", TensorProto.FLOAT, [None, None])
C = make_tensor_value_info("C", to, [None, None])
if cuda:
nodes = [
make_node("Cast", ["A"], ["Cc"], to=to),
make_node("MemcpyFromHost", ["Cc"], ["C"]),
]
else:
nodes = [make_node("Cast", ["A"], ["C"], to=to)]
graph = make_graph(nodes, "a", [A], [C])
if to < 16:
# regular type
opset, ir = 18, 8
else:
opset, ir = 19, 9
onnx_model = make_model(
graph, opset_imports=[make_opsetid("", opset)], ir_version=ir
)
if not cuda:
# OpType: MemcpyFromHost
check_model(onnx_model)
return onnx_model
print(onnx_simple_text_plot(create_cast(TensorProto.FLOAT16)))
opset: domain='' version=18
input: name='A' type=dtype('float32') shape=['', '']
Cast(A, to=10) -> C
output: name='C' type=dtype('float16') shape=['', '']
Performance#
The benchmark will run the following configurations.
types = list(getattr(TensorProto, a) for a in script_args.types.split(","))
engine = [InferenceSession, CReferenceEvaluator]
providers = [
["CUDAExecutionProvider", "CPUExecutionProvider"],
["CPUExecutionProvider"],
]
# M, N, K
# we use multiple of 8, otherwise, float8 does not work.
dims = [list(int(i) for i in line.split(",")) for line in script_args.dims.split(";")]
domains = ["onnx_extented.ortops.tutorial.cuda", "", "com.microsoft"]
Let’s cache the matrices involved.
def to_ort_value(m):
device = C_OrtDevice(C_OrtDevice.cpu(), C_OrtDevice.default_memory(), 0)
ort_value = C_OrtValue.ortvalue_from_numpy(m, device)
return ort_value
def cached_inputs(dims, types):
matrices = {}
matrices_cuda = {}
pbar = tqdm(list(product(dims, types)))
for dim, tt in pbar:
m, n, k = dim
pbar.set_description(f"t={tt} dim={dim}")
for i, j in [(m, k), (k, n), (k, m)]:
if (tt, i, j) in matrices:
continue
# CPU
try:
sess = InferenceSession(
create_cast(tt).SerializeToString(),
providers=["CPUExecutionProvider"],
)
cpu = True
except (InvalidGraph, InvalidArgument, NotImplemented):
# not support by this version of onnxruntime
cpu = False
if cpu:
vect = (numpy.random.randn(i, j) * 10).astype(numpy.float32)
ov = to_ort_value(vect)
ovtt = sess._sess.run_with_ort_values({"A": ov}, ["C"], None)[0]
matrices[tt, i, j] = ovtt
else:
continue
# CUDA
if "CUDAExecutionProvider" not in get_available_providers():
# No CUDA
continue
sess = InferenceSession(
create_cast(tt, cuda=True).SerializeToString(),
providers=["CUDAExecutionProvider", "CPUExecutionProvider"],
)
vect = (numpy.random.randn(i, j) * 10).astype(numpy.float32)
ov = to_ort_value(vect)
ovtt = sess._sess.run_with_ort_values({"A": ov}, ["C"], None)[0]
matrices_cuda[tt, i, j] = ovtt
return matrices, matrices_cuda
matrices, matrices_cuda = cached_inputs(dims, types)
print(f"{len(matrices)} matrices were created.")
0%| | 0/28 [00:00<?, ?it/s]
t=17 dim=[32, 32, 32]: 0%| | 0/28 [00:00<?, ?it/s]
t=17 dim=[32, 32, 32]: 4%|▎ | 1/28 [00:20<09:10, 20.40s/it]
t=1 dim=[32, 32, 32]: 4%|▎ | 1/28 [00:20<09:10, 20.40s/it]
t=10 dim=[32, 32, 32]: 4%|▎ | 1/28 [00:20<09:10, 20.40s/it]
t=16 dim=[32, 32, 32]: 4%|▎ | 1/28 [00:20<09:10, 20.40s/it]
t=17 dim=[64, 64, 64]: 4%|▎ | 1/28 [00:20<09:10, 20.40s/it]
t=1 dim=[64, 64, 64]: 4%|▎ | 1/28 [00:20<09:10, 20.40s/it]
t=1 dim=[64, 64, 64]: 21%|██▏ | 6/28 [00:20<00:55, 2.53s/it]
t=10 dim=[64, 64, 64]: 21%|██▏ | 6/28 [00:20<00:55, 2.53s/it]
t=16 dim=[64, 64, 64]: 21%|██▏ | 6/28 [00:20<00:55, 2.53s/it]
t=17 dim=[128, 128, 128]: 21%|██▏ | 6/28 [00:20<00:55, 2.53s/it]
t=1 dim=[128, 128, 128]: 21%|██▏ | 6/28 [00:20<00:55, 2.53s/it]
t=10 dim=[128, 128, 128]: 21%|██▏ | 6/28 [00:20<00:55, 2.53s/it]
t=10 dim=[128, 128, 128]: 39%|███▉ | 11/28 [00:20<00:19, 1.14s/it]
t=16 dim=[128, 128, 128]: 39%|███▉ | 11/28 [00:20<00:19, 1.14s/it]
t=17 dim=[256, 256, 256]: 39%|███▉ | 11/28 [00:20<00:19, 1.14s/it]
t=1 dim=[256, 256, 256]: 39%|███▉ | 11/28 [00:20<00:19, 1.14s/it]
t=10 dim=[256, 256, 256]: 39%|███▉ | 11/28 [00:20<00:19, 1.14s/it]
t=10 dim=[256, 256, 256]: 54%|█████▎ | 15/28 [00:21<00:09, 1.37it/s]
t=16 dim=[256, 256, 256]: 54%|█████▎ | 15/28 [00:21<00:09, 1.37it/s]
t=17 dim=[400, 400, 400]: 54%|█████▎ | 15/28 [00:21<00:09, 1.37it/s]
t=1 dim=[400, 400, 400]: 54%|█████▎ | 15/28 [00:21<00:09, 1.37it/s]
t=1 dim=[400, 400, 400]: 64%|██████▍ | 18/28 [00:21<00:05, 1.82it/s]
t=10 dim=[400, 400, 400]: 64%|██████▍ | 18/28 [00:21<00:05, 1.82it/s]
t=16 dim=[400, 400, 400]: 64%|██████▍ | 18/28 [00:21<00:05, 1.82it/s]
t=16 dim=[400, 400, 400]: 71%|███████▏ | 20/28 [00:21<00:03, 2.20it/s]
t=17 dim=[512, 512, 512]: 71%|███████▏ | 20/28 [00:21<00:03, 2.20it/s]
t=1 dim=[512, 512, 512]: 71%|███████▏ | 20/28 [00:21<00:03, 2.20it/s]
t=1 dim=[512, 512, 512]: 79%|███████▊ | 22/28 [00:21<00:02, 2.64it/s]
t=10 dim=[512, 512, 512]: 79%|███████▊ | 22/28 [00:21<00:02, 2.64it/s]
t=16 dim=[512, 512, 512]: 79%|███████▊ | 22/28 [00:22<00:02, 2.64it/s]
t=16 dim=[512, 512, 512]: 86%|████████▌ | 24/28 [00:22<00:01, 3.18it/s]
t=17 dim=[1024, 1024, 1024]: 86%|████████▌ | 24/28 [00:22<00:01, 3.18it/s]
t=1 dim=[1024, 1024, 1024]: 86%|████████▌ | 24/28 [00:22<00:01, 3.18it/s]
t=1 dim=[1024, 1024, 1024]: 93%|█████████▎| 26/28 [00:22<00:00, 3.25it/s]
t=10 dim=[1024, 1024, 1024]: 93%|█████████▎| 26/28 [00:22<00:00, 3.25it/s]
t=10 dim=[1024, 1024, 1024]: 96%|█████████▋| 27/28 [00:22<00:00, 3.47it/s]
t=16 dim=[1024, 1024, 1024]: 96%|█████████▋| 27/28 [00:22<00:00, 3.47it/s]
t=16 dim=[1024, 1024, 1024]: 100%|██████████| 28/28 [00:23<00:00, 3.69it/s]
t=16 dim=[1024, 1024, 1024]: 100%|██████████| 28/28 [00:23<00:00, 1.21it/s]
28 matrices were created.
Let’s run the benchmark
def rendering_obs(obs, dim, number, repeat, domain, provider, internal_time):
stype = {
TensorProto.FLOAT: "f32",
TensorProto.FLOAT16: "f16",
TensorProto.BFLOAT16: "bf16",
TensorProto.INT8: "i8",
TensorProto.INT16: "i16",
TensorProto.INT32: "i32",
TensorProto.UINT32: "u32",
TensorProto.FLOAT8E4M3FN: "e4m3fn",
TensorProto.FLOAT8E5M2: "e5m2",
}[tt]
obs.update(
dict(
engine={"InferenceSession": "ort", "CReferenceEvaluator": "np"}[
engine.__name__
],
stype=stype,
type=f"{stype}",
M=dim[0],
N=dim[1],
K=dim[2],
cost=numpy.prod(dim) * 4,
cost_s=f"{numpy.prod(dim) * 4}-{dim[0]}x{dim[1]}x{dim[2]}",
repeat=repeat,
number=number,
domain={
"": "ORT",
"com.microsoft": "COM",
"onnx_extented.ortops.tutorial.cuda": "EXT",
}[domain],
provider={
"CPUExecutionProvider": "cpu",
"CUDAExecutionProvider": "cuda",
}[provider[0]],
platform=platform.processor(),
intime=internal_time,
)
)
return obs
opts = SessionOptions()
r = get_ort_ext_libs()
if r is not None:
opts.register_custom_ops_library(r[0])
data = []
errors = []
pbar = tqdm(list(product(types, engine, providers, dims, domains)))
for tt, engine, provider, dim, domain in pbar:
if (
tt in {TensorProto.FLOAT8E4M3FN, TensorProto.FLOAT8E5M2}
and properties.get("major", 0) < 9
):
# f8 not available
if provider[0] == "CPUExecutionProvider":
continue
errors.append(
f"f8 not available, major={properties.get('major', 0)}, "
f"tt={tt}, provider={provider!r}, domain={domain!r}."
)
continue
elif provider[0] == "CPUExecutionProvider" and max(dim) > 2000:
# too long
continue
if max(dim) <= 200:
repeat, number = script_args.repeat * 4, script_args.number * 4
elif max(dim) <= 256:
repeat, number = script_args.repeat * 2, script_args.number * 2
else:
repeat, number = script_args.repeat, script_args.number
onx = create_model(tt, provider=provider[0], domain=domain)
if onx is None:
if provider[0] == "CPUExecutionProvider":
continue
errors.append(
f"No model for tt={tt}, provider={provider!r}, domain={domain!r}."
)
continue
with open(f"plot_bench_gemm_ort_{tt}_{domain}.onnx", "wb") as f:
f.write(onx.SerializeToString())
k1 = (tt, dim[2], dim[0])
k2 = (tt, dim[2], dim[1])
if k1 not in matrices:
errors.append(f"Key k1={k1!r} not in matrices.")
continue
if k2 not in matrices:
errors.append(f"Key k2={k2!r} not in matrices.")
continue
pbar.set_description(f"t={tt} e={engine.__name__} p={provider[0][:4]} dim={dim}")
if engine == CReferenceEvaluator:
if (
domain != ""
or max(dim) > 256
or provider != ["CPUExecutionProvider"]
or tt not in [TensorProto.FLOAT, TensorProto.FLOAT16]
):
# All impossible or slow cases.
continue
if tt == TensorProto.FLOAT16 and max(dim) > 50:
repeat, number = 2, 2
feeds = {"A": matrices[k1].numpy(), "B": matrices[k2].numpy()}
sess = engine(onx)
sess.run(None, feeds)
obs = measure_time(lambda: sess.run(None, feeds), repeat=repeat, number=number)
elif engine == InferenceSession:
if provider[0] not in get_available_providers():
errors.append(f"provider={provider[0]} is missing")
continue
try:
sess = engine(onx.SerializeToString(), opts, providers=provider)
except (NotImplemented, InvalidGraph, Fail) as e:
# not implemented
errors.append((tt, engine.__class__.__name__, provider, domain, e))
continue
the_feeds = (
{"A": matrices[k1], "B": matrices[k2]}
if provider == ["CPUExecutionProvider"]
else {"A": matrices_cuda[k1], "B": matrices_cuda[k2]}
)
out_names = ["C"]
# warmup
for i in range(script_args.warmup):
sess._sess.run_with_ort_values(the_feeds, out_names, None)[0]
# benchamrk
times = []
def fct_benchmarked():
got = sess._sess.run_with_ort_values(the_feeds, out_names, None)
if len(got) > 1:
times.append(got[1])
obs = measure_time(fct_benchmarked, repeat=repeat, number=number)
internal_time = None
if len(times) > 0:
np_times = [t.numpy() for t in times]
internal_time = (sum(np_times) / len(times))[0]
else:
errors.append(f"unknown engine={engine}")
continue
# improves the rendering
obs = rendering_obs(obs, dim, number, repeat, domain, provider, internal_time)
data.append(obs)
if unit_test_going() and len(data) >= 2:
break
0%| | 0/336 [00:00<?, ?it/s]
t=1 e=InferenceSession p=CUDA dim=[32, 32, 32]: 0%| | 0/336 [00:00<?, ?it/s]
t=1 e=InferenceSession p=CUDA dim=[32, 32, 32]: 25%|██▌ | 85/336 [00:07<00:22, 10.94it/s]
t=1 e=InferenceSession p=CUDA dim=[32, 32, 32]: 25%|██▌ | 85/336 [00:07<00:22, 10.94it/s]
t=1 e=InferenceSession p=CUDA dim=[32, 32, 32]: 25%|██▌ | 85/336 [00:08<00:22, 10.94it/s]
t=1 e=InferenceSession p=CUDA dim=[32, 32, 32]: 26%|██▌ | 87/336 [00:08<00:23, 10.48it/s]
t=1 e=InferenceSession p=CUDA dim=[64, 64, 64]: 26%|██▌ | 87/336 [00:08<00:23, 10.48it/s]
t=1 e=InferenceSession p=CUDA dim=[64, 64, 64]: 26%|██▌ | 88/336 [00:13<00:50, 4.92it/s]
t=1 e=InferenceSession p=CUDA dim=[64, 64, 64]: 26%|██▌ | 88/336 [00:13<00:50, 4.92it/s]
t=1 e=InferenceSession p=CUDA dim=[64, 64, 64]: 26%|██▋ | 89/336 [00:13<00:50, 4.88it/s]
t=1 e=InferenceSession p=CUDA dim=[64, 64, 64]: 26%|██▋ | 89/336 [00:13<00:50, 4.88it/s]
t=1 e=InferenceSession p=CUDA dim=[128, 128, 128]: 26%|██▋ | 89/336 [00:13<00:50, 4.88it/s]
t=1 e=InferenceSession p=CUDA dim=[128, 128, 128]: 27%|██▋ | 91/336 [00:18<01:39, 2.45it/s]
t=1 e=InferenceSession p=CUDA dim=[128, 128, 128]: 27%|██▋ | 91/336 [00:18<01:39, 2.45it/s]
t=1 e=InferenceSession p=CUDA dim=[128, 128, 128]: 27%|██▋ | 92/336 [00:19<01:40, 2.44it/s]
t=1 e=InferenceSession p=CUDA dim=[128, 128, 128]: 27%|██▋ | 92/336 [00:19<01:40, 2.44it/s]
t=1 e=InferenceSession p=CUDA dim=[256, 256, 256]: 27%|██▋ | 92/336 [00:19<01:40, 2.44it/s]
t=1 e=InferenceSession p=CUDA dim=[256, 256, 256]: 28%|██▊ | 94/336 [00:20<01:49, 2.21it/s]
t=1 e=InferenceSession p=CUDA dim=[256, 256, 256]: 28%|██▊ | 94/336 [00:20<01:49, 2.21it/s]
t=1 e=InferenceSession p=CUDA dim=[256, 256, 256]: 28%|██▊ | 95/336 [00:20<01:46, 2.26it/s]
t=1 e=InferenceSession p=CUDA dim=[256, 256, 256]: 28%|██▊ | 95/336 [00:20<01:46, 2.26it/s]
t=1 e=InferenceSession p=CUDA dim=[400, 400, 400]: 28%|██▊ | 95/336 [00:20<01:46, 2.26it/s]
t=1 e=InferenceSession p=CUDA dim=[400, 400, 400]: 29%|██▉ | 97/336 [00:21<01:37, 2.46it/s]
t=1 e=InferenceSession p=CUDA dim=[400, 400, 400]: 29%|██▉ | 97/336 [00:21<01:37, 2.46it/s]
t=1 e=InferenceSession p=CUDA dim=[400, 400, 400]: 29%|██▉ | 98/336 [00:21<01:30, 2.62it/s]
t=1 e=InferenceSession p=CUDA dim=[400, 400, 400]: 29%|██▉ | 98/336 [00:21<01:30, 2.62it/s]
t=1 e=InferenceSession p=CUDA dim=[512, 512, 512]: 29%|██▉ | 98/336 [00:21<01:30, 2.62it/s]
t=1 e=InferenceSession p=CUDA dim=[512, 512, 512]: 30%|██▉ | 100/336 [00:22<01:25, 2.75it/s]
t=1 e=InferenceSession p=CUDA dim=[512, 512, 512]: 30%|██▉ | 100/336 [00:22<01:25, 2.75it/s]
t=1 e=InferenceSession p=CUDA dim=[512, 512, 512]: 30%|███ | 101/336 [00:22<01:25, 2.76it/s]
t=1 e=InferenceSession p=CUDA dim=[512, 512, 512]: 30%|███ | 101/336 [00:22<01:25, 2.76it/s]
t=1 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 30%|███ | 101/336 [00:22<01:25, 2.76it/s]
t=1 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 31%|███ | 103/336 [00:24<02:06, 1.84it/s]
t=1 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 31%|███ | 103/336 [00:24<02:06, 1.84it/s]
t=1 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 31%|███ | 104/336 [00:25<02:42, 1.43it/s]
t=1 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 31%|███ | 104/336 [00:25<02:42, 1.43it/s]
t=1 e=InferenceSession p=CPUE dim=[32, 32, 32]: 31%|███ | 104/336 [00:25<02:42, 1.43it/s]
t=1 e=InferenceSession p=CPUE dim=[64, 64, 64]: 31%|███ | 104/336 [00:25<02:42, 1.43it/s]
t=1 e=InferenceSession p=CPUE dim=[128, 128, 128]: 31%|███ | 104/336 [00:25<02:42, 1.43it/s]
t=1 e=InferenceSession p=CPUE dim=[256, 256, 256]: 31%|███ | 104/336 [00:25<02:42, 1.43it/s]
t=1 e=InferenceSession p=CPUE dim=[256, 256, 256]: 35%|███▍ | 116/336 [00:26<00:39, 5.61it/s]
t=1 e=InferenceSession p=CPUE dim=[400, 400, 400]: 35%|███▍ | 116/336 [00:26<00:39, 5.61it/s]
t=1 e=InferenceSession p=CPUE dim=[512, 512, 512]: 35%|███▍ | 116/336 [00:26<00:39, 5.61it/s]
t=1 e=InferenceSession p=CPUE dim=[512, 512, 512]: 36%|███▋ | 122/336 [00:26<00:26, 7.97it/s]
t=1 e=InferenceSession p=CPUE dim=[1024, 1024, 1024]: 36%|███▋ | 122/336 [00:26<00:26, 7.97it/s]
t=1 e=InferenceSession p=CPUE dim=[1024, 1024, 1024]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[32, 32, 32]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[64, 64, 64]: 37%|███▋ | 125/336 [00:27<00:34, 6.11it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[64, 64, 64]: 45%|████▌ | 152/336 [00:27<00:09, 20.41it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[128, 128, 128]: 45%|████▌ | 152/336 [00:27<00:09, 20.41it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[256, 256, 256]: 45%|████▌ | 152/336 [00:28<00:09, 20.41it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[400, 400, 400]: 45%|████▌ | 152/336 [00:28<00:09, 20.41it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[400, 400, 400]: 48%|████▊ | 161/336 [00:28<00:12, 13.95it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[512, 512, 512]: 48%|████▊ | 161/336 [00:28<00:12, 13.95it/s]
t=1 e=CReferenceEvaluator p=CPUE dim=[1024, 1024, 1024]: 48%|████▊ | 161/336 [00:28<00:12, 13.95it/s]
t=10 e=InferenceSession p=CUDA dim=[32, 32, 32]: 48%|████▊ | 161/336 [00:28<00:12, 13.95it/s]
t=10 e=InferenceSession p=CUDA dim=[32, 32, 32]: 50%|█████ | 169/336 [00:35<00:46, 3.62it/s]
t=10 e=InferenceSession p=CUDA dim=[32, 32, 32]: 50%|█████ | 169/336 [00:35<00:46, 3.62it/s]
t=10 e=InferenceSession p=CUDA dim=[32, 32, 32]: 50%|█████ | 169/336 [00:36<00:46, 3.62it/s]
t=10 e=InferenceSession p=CUDA dim=[64, 64, 64]: 50%|█████ | 169/336 [00:36<00:46, 3.62it/s]
t=10 e=InferenceSession p=CUDA dim=[64, 64, 64]: 50%|█████ | 169/336 [00:44<00:46, 3.62it/s]
t=10 e=InferenceSession p=CUDA dim=[64, 64, 64]: 50%|█████ | 169/336 [00:44<00:46, 3.62it/s]
t=10 e=InferenceSession p=CUDA dim=[64, 64, 64]: 52%|█████▏ | 174/336 [00:44<01:29, 1.80it/s]
t=10 e=InferenceSession p=CUDA dim=[128, 128, 128]: 52%|█████▏ | 174/336 [00:44<01:29, 1.80it/s]
t=10 e=InferenceSession p=CUDA dim=[128, 128, 128]: 52%|█████▏ | 174/336 [00:53<01:29, 1.80it/s]
t=10 e=InferenceSession p=CUDA dim=[128, 128, 128]: 52%|█████▏ | 174/336 [00:53<01:29, 1.80it/s]
t=10 e=InferenceSession p=CUDA dim=[128, 128, 128]: 53%|█████▎ | 177/336 [00:53<02:18, 1.15it/s]
t=10 e=InferenceSession p=CUDA dim=[256, 256, 256]: 53%|█████▎ | 177/336 [00:53<02:18, 1.15it/s]
t=10 e=InferenceSession p=CUDA dim=[256, 256, 256]: 53%|█████▎ | 177/336 [00:56<02:18, 1.15it/s]
t=10 e=InferenceSession p=CUDA dim=[256, 256, 256]: 53%|█████▎ | 177/336 [00:57<02:18, 1.15it/s]
t=10 e=InferenceSession p=CUDA dim=[256, 256, 256]: 54%|█████▎ | 180/336 [00:57<02:23, 1.08it/s]
t=10 e=InferenceSession p=CUDA dim=[400, 400, 400]: 54%|█████▎ | 180/336 [00:57<02:23, 1.08it/s]
t=10 e=InferenceSession p=CUDA dim=[400, 400, 400]: 54%|█████▎ | 180/336 [01:00<02:23, 1.08it/s]
t=10 e=InferenceSession p=CUDA dim=[400, 400, 400]: 54%|█████▍ | 182/336 [01:00<02:38, 1.03s/it]
t=10 e=InferenceSession p=CUDA dim=[400, 400, 400]: 54%|█████▍ | 182/336 [01:00<02:38, 1.03s/it]
t=10 e=InferenceSession p=CUDA dim=[512, 512, 512]: 54%|█████▍ | 182/336 [01:00<02:38, 1.03s/it]
t=10 e=InferenceSession p=CUDA dim=[512, 512, 512]: 55%|█████▍ | 184/336 [01:06<03:16, 1.29s/it]
t=10 e=InferenceSession p=CUDA dim=[512, 512, 512]: 55%|█████▍ | 184/336 [01:06<03:16, 1.29s/it]
t=10 e=InferenceSession p=CUDA dim=[512, 512, 512]: 55%|█████▌ | 185/336 [01:06<02:58, 1.18s/it]
t=10 e=InferenceSession p=CUDA dim=[512, 512, 512]: 55%|█████▌ | 185/336 [01:06<02:58, 1.18s/it]
t=10 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 55%|█████▌ | 185/336 [01:06<02:58, 1.18s/it]
t=10 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 56%|█████▌ | 187/336 [01:40<11:56, 4.81s/it]
t=10 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 56%|█████▌ | 187/336 [01:40<11:56, 4.81s/it]
t=10 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 56%|█████▌ | 188/336 [01:41<10:33, 4.28s/it]
t=10 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 56%|█████▌ | 188/336 [01:41<10:33, 4.28s/it]
t=10 e=InferenceSession p=CPUE dim=[32, 32, 32]: 56%|█████▌ | 188/336 [01:41<10:33, 4.28s/it]
t=10 e=InferenceSession p=CPUE dim=[64, 64, 64]: 56%|█████▌ | 188/336 [01:41<10:33, 4.28s/it]
t=10 e=InferenceSession p=CPUE dim=[128, 128, 128]: 56%|█████▌ | 188/336 [01:41<10:33, 4.28s/it]
t=10 e=InferenceSession p=CPUE dim=[128, 128, 128]: 59%|█████▊ | 197/336 [01:42<03:36, 1.55s/it]
t=10 e=InferenceSession p=CPUE dim=[256, 256, 256]: 59%|█████▊ | 197/336 [01:42<03:36, 1.55s/it]
t=10 e=InferenceSession p=CPUE dim=[256, 256, 256]: 60%|█████▉ | 200/336 [01:42<02:43, 1.21s/it]
t=10 e=InferenceSession p=CPUE dim=[400, 400, 400]: 60%|█████▉ | 200/336 [01:42<02:43, 1.21s/it]
t=10 e=InferenceSession p=CPUE dim=[400, 400, 400]: 60%|██████ | 203/336 [01:42<02:02, 1.09it/s]
t=10 e=InferenceSession p=CPUE dim=[512, 512, 512]: 60%|██████ | 203/336 [01:42<02:02, 1.09it/s]
t=10 e=InferenceSession p=CPUE dim=[512, 512, 512]: 61%|██████▏ | 206/336 [01:42<01:31, 1.43it/s]
t=10 e=InferenceSession p=CPUE dim=[1024, 1024, 1024]: 61%|██████▏ | 206/336 [01:42<01:31, 1.43it/s]
t=10 e=InferenceSession p=CPUE dim=[1024, 1024, 1024]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[32, 32, 32]: 62%|██████▏ | 209/336 [01:44<01:19, 1.59it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[32, 32, 32]: 69%|██████▉ | 233/336 [01:44<00:16, 6.23it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[64, 64, 64]: 69%|██████▉ | 233/336 [01:44<00:16, 6.23it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[128, 128, 128]: 69%|██████▉ | 233/336 [01:44<00:16, 6.23it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[256, 256, 256]: 69%|██████▉ | 233/336 [01:44<00:16, 6.23it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[256, 256, 256]: 72%|███████▏ | 242/336 [01:45<00:13, 7.20it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[400, 400, 400]: 72%|███████▏ | 242/336 [01:45<00:13, 7.20it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[512, 512, 512]: 72%|███████▏ | 242/336 [01:45<00:13, 7.20it/s]
t=10 e=CReferenceEvaluator p=CPUE dim=[1024, 1024, 1024]: 72%|███████▏ | 242/336 [01:45<00:13, 7.20it/s]
t=16 e=InferenceSession p=CUDA dim=[32, 32, 32]: 72%|███████▏ | 242/336 [01:45<00:13, 7.20it/s]
t=16 e=InferenceSession p=CUDA dim=[32, 32, 32]: 76%|███████▌ | 254/336 [01:45<00:08, 9.93it/s]
t=16 e=InferenceSession p=CUDA dim=[32, 32, 32]: 76%|███████▌ | 254/336 [01:45<00:08, 9.93it/s]
t=16 e=InferenceSession p=CUDA dim=[64, 64, 64]: 76%|███████▌ | 254/336 [01:45<00:08, 9.93it/s]
t=16 e=InferenceSession p=CUDA dim=[64, 64, 64]: 76%|███████▋ | 257/336 [01:45<00:08, 9.78it/s]
t=16 e=InferenceSession p=CUDA dim=[64, 64, 64]: 76%|███████▋ | 257/336 [01:45<00:08, 9.78it/s]
t=16 e=InferenceSession p=CUDA dim=[128, 128, 128]: 76%|███████▋ | 257/336 [01:45<00:08, 9.78it/s]
t=16 e=InferenceSession p=CUDA dim=[128, 128, 128]: 77%|███████▋ | 260/336 [01:46<00:08, 9.08it/s]
t=16 e=InferenceSession p=CUDA dim=[128, 128, 128]: 77%|███████▋ | 260/336 [01:46<00:08, 9.08it/s]
t=16 e=InferenceSession p=CUDA dim=[256, 256, 256]: 77%|███████▋ | 260/336 [01:46<00:08, 9.08it/s]
t=16 e=InferenceSession p=CUDA dim=[256, 256, 256]: 78%|███████▊ | 263/336 [01:46<00:08, 9.12it/s]
t=16 e=InferenceSession p=CUDA dim=[256, 256, 256]: 78%|███████▊ | 263/336 [01:46<00:08, 9.12it/s]
t=16 e=InferenceSession p=CUDA dim=[400, 400, 400]: 78%|███████▊ | 263/336 [01:46<00:08, 9.12it/s]
t=16 e=InferenceSession p=CUDA dim=[400, 400, 400]: 79%|███████▉ | 266/336 [01:46<00:07, 9.79it/s]
t=16 e=InferenceSession p=CUDA dim=[400, 400, 400]: 79%|███████▉ | 266/336 [01:46<00:07, 9.79it/s]
t=16 e=InferenceSession p=CUDA dim=[512, 512, 512]: 79%|███████▉ | 266/336 [01:46<00:07, 9.79it/s]
t=16 e=InferenceSession p=CUDA dim=[512, 512, 512]: 80%|████████ | 269/336 [01:47<00:06, 9.92it/s]
t=16 e=InferenceSession p=CUDA dim=[512, 512, 512]: 80%|████████ | 269/336 [01:47<00:06, 9.92it/s]
t=16 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 80%|████████ | 269/336 [01:47<00:06, 9.92it/s]
t=16 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CUDA dim=[1024, 1024, 1024]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CPUE dim=[32, 32, 32]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CPUE dim=[64, 64, 64]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CPUE dim=[128, 128, 128]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CPUE dim=[256, 256, 256]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CPUE dim=[400, 400, 400]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CPUE dim=[512, 512, 512]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=InferenceSession p=CPUE dim=[1024, 1024, 1024]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[32, 32, 32]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[64, 64, 64]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[128, 128, 128]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[256, 256, 256]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[400, 400, 400]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[512, 512, 512]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CUDA dim=[1024, 1024, 1024]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[32, 32, 32]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[64, 64, 64]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[128, 128, 128]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[256, 256, 256]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[400, 400, 400]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[512, 512, 512]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[1024, 1024, 1024]: 81%|████████ | 272/336 [01:48<00:11, 5.65it/s]
t=16 e=CReferenceEvaluator p=CPUE dim=[1024, 1024, 1024]: 100%|██████████| 336/336 [01:48<00:00, 3.10it/s]
Results#
0 ... 4
average 0.009009 ... 0.008234
deviation 0.002026 ... 0.001375
min_exec 0.005501 ... 0.005689
max_exec 0.012335 ... 0.010571
repeat 40 ... 40
number 16 ... 16
ttime 0.360375 ... 0.329349
context_size 64 ... 64
warmup_time 0.00644 ... 0.006481
engine ort ... ort
stype f32 ... f32
type f32 ... f32
M 32 ... 128
N 32 ... 128
K 32 ... 128
cost 131072 ... 8388608
cost_s 131072-32x32x32 ... 8388608-128x128x128
domain EXT ... EXT
provider cuda ... cuda
platform x86_64 ... x86_64
intime None ... None
[21 rows x 5 columns]
The errors#
1/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
2/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
3/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
4/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
5/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
6/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
7/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
8/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
9/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
10/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
11/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
12/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
13/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
14/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
15/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
16/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
17/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
18/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
19/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
20/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
21/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
22/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
23/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
24/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
25/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
26/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
27/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
28/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
29/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
30/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
31/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
32/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
33/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
34/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
35/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
36/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
37/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
38/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
39/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
40/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
41/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain=''.
42/84-f8 not available, major=6, tt=17, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='com.microsoft'.
43/84-(1, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
44/84-(1, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
45/84-(1, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
46/84-(1, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
47/84-(1, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
48/84-(1, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
49/84-(1, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
50/84-(10, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
51/84-(10, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
52/84-(10, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
53/84-(10, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
54/84-(10, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
55/84-(10, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
56/84-(10, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
57/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
58/84-(16, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
59/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
60/84-(16, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
61/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
62/84-(16, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
63/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
64/84-(16, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
65/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
66/84-(16, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
67/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
68/84-(16, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
69/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
70/84-(16, 'type', ['CUDAExecutionProvider', 'CPUExecutionProvider'], 'com.microsoft', Fail('[ONNXRuntimeError] : 1 : FAIL : Fatal error: com.microsoft:GemmFloat8(-1) is not a registered function/op'))
71/84-(16, 'type', ['CPUExecutionProvider'], '', NotImplemented("[ONNXRuntimeError] : 9 : NOT_IMPLEMENTED : Could not find an implementation for Gemm(13) node with name ''"))
72/84-(16, 'type', ['CPUExecutionProvider'], '', NotImplemented("[ONNXRuntimeError] : 9 : NOT_IMPLEMENTED : Could not find an implementation for Gemm(13) node with name ''"))
73/84-(16, 'type', ['CPUExecutionProvider'], '', NotImplemented("[ONNXRuntimeError] : 9 : NOT_IMPLEMENTED : Could not find an implementation for Gemm(13) node with name ''"))
74/84-(16, 'type', ['CPUExecutionProvider'], '', NotImplemented("[ONNXRuntimeError] : 9 : NOT_IMPLEMENTED : Could not find an implementation for Gemm(13) node with name ''"))
75/84-(16, 'type', ['CPUExecutionProvider'], '', NotImplemented("[ONNXRuntimeError] : 9 : NOT_IMPLEMENTED : Could not find an implementation for Gemm(13) node with name ''"))
76/84-(16, 'type', ['CPUExecutionProvider'], '', NotImplemented("[ONNXRuntimeError] : 9 : NOT_IMPLEMENTED : Could not find an implementation for Gemm(13) node with name ''"))
77/84-(16, 'type', ['CPUExecutionProvider'], '', NotImplemented("[ONNXRuntimeError] : 9 : NOT_IMPLEMENTED : Could not find an implementation for Gemm(13) node with name ''"))
78/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
79/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
80/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
81/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
82/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
83/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
84/84-No model for tt=16, provider=['CUDAExecutionProvider', 'CPUExecutionProvider'], domain='onnx_extented.ortops.tutorial.cuda'.
Summary#
piv = pivot_table(
df,
index=["cost"],
columns=["provider", "type", "domain", "engine"],
values=["average", "intime"],
)
piv.reset_index(drop=False).to_excel("plot_bench_gemm_ort_summary.xlsx")
piv.reset_index(drop=False).to_csv("plot_bench_gemm_ort_summary.csv")
print("summary")
print(piv)
piv
summary
average ...
provider cpu ... cuda
type f16 f32 ... f16 f32
domain ORT ORT ... ORT EXT ORT
engine np ort np ... ort ort ort
cost ...
131072 0.000370 0.000042 0.000051 ... 0.000407 0.009009 0.000609
1048576 0.001747 0.000060 0.000060 ... 0.000377 0.007497 0.000374
8388608 0.011787 0.000233 0.001453 ... 0.000553 0.008234 0.000690
67108864 0.110748 0.000995 0.001599 ... 0.001673 0.008488 0.001935
256000000 NaN 0.003304 NaN ... 0.003287 0.011320 0.004401
536870912 NaN 0.006177 NaN ... 0.004559 0.013148 0.007626
4294967296 NaN 0.027700 NaN ... 0.021694 0.040819 0.029408
[7 rows x 9 columns]
With the dimensions.
pivs = pivot_table(
df,
index=["cost_s"],
columns=["provider", "type", "domain", "engine"],
values=["average", "intime"],
)
print(pivs)
average ...
provider cpu ... cuda
type f16 ... f32
domain ORT ... EXT ORT
engine np ort ... ort ort
cost_s ...
1048576-64x64x64 0.001747 0.000060 ... 0.007497 0.000374
131072-32x32x32 0.000370 0.000042 ... 0.009009 0.000609
256000000-400x400x400 NaN 0.003304 ... 0.011320 0.004401
4294967296-1024x1024x1024 NaN 0.027700 ... 0.040819 0.029408
536870912-512x512x512 NaN 0.006177 ... 0.013148 0.007626
67108864-256x256x256 0.110748 0.000995 ... 0.008488 0.001935
8388608-128x128x128 0.011787 0.000233 ... 0.008234 0.000690
[7 rows x 9 columns]
plot
dfi = df[
df.type.isin({"f32", "f16", "bf16", "e4m3fn", "e5m2"}) & df.engine.isin({"ort"})
]
pivi = pivot_table(
dfi,
index=["cost"],
columns=["type", "domain", "provider", "engine"],
values="average",
)
fig, ax = plt.subplots(1, 2, figsize=(12, 6))
piv.plot(ax=ax[0], title="Gemm performance\nlower is better", logx=True, logy=True)
if pivi.shape[0] > 0:
pivi.plot(
ax=ax[1],
title=f"Gemm performance ORT\n{platform.processor()}",
logx=True,
logy=True,
)
fig.tight_layout()
fig.savefig("plot_bench_gemm_ort.png")
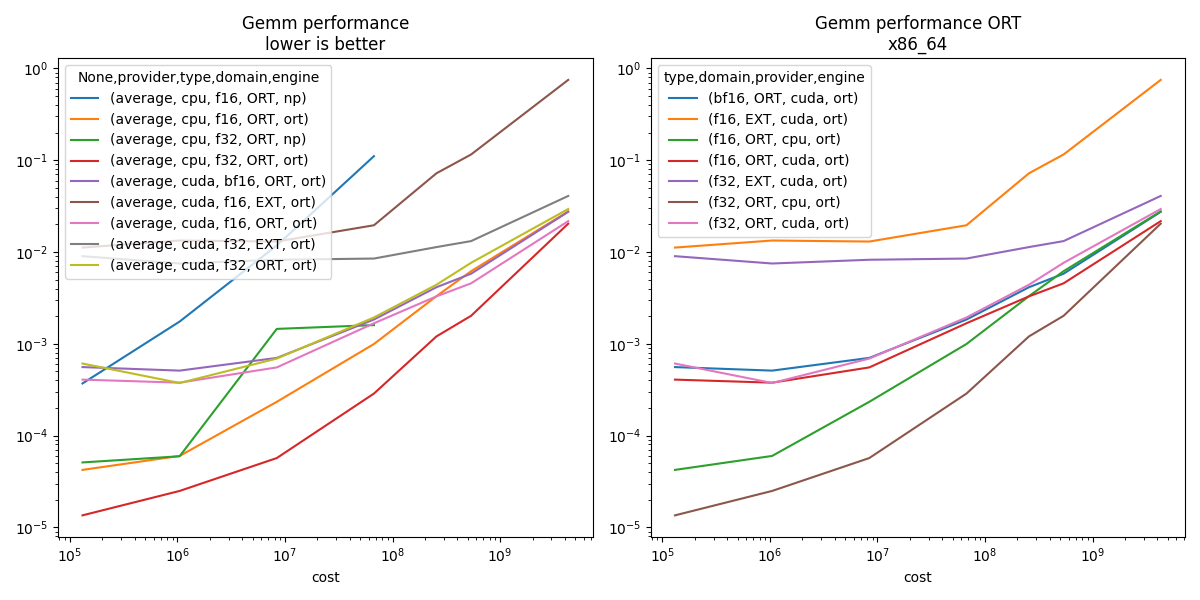
2023-09-16 12:25:18,218 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,218 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,234 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,235 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,237 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,238 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,372 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,372 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,373 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,373 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,374 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,374 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,375 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,375 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,379 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,379 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,380 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,380 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,398 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,399 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,408 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,408 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,409 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,409 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,491 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,491 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,492 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,492 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,493 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,493 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,493 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,494 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,497 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,497 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,498 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,498 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,500 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,500 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,501 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,501 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,502 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,502 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,506 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,506 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,507 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,507 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,508 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,508 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,535 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,535 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,536 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,536 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,537 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,537 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,548 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,548 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,558 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,558 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,559 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,559 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,719 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,719 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,721 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,721 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,723 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,723 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,789 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,789 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,791 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,791 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,793 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,793 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,814 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,814 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,817 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,818 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,820 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,820 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,828 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,828 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,829 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,829 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,831 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,832 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,852 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,852 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,853 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,854 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,856 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,856 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,884 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,884 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,886 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,886 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,888 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,889 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,897 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,897 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,900 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,900 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:18,902 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:18,902 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:18,914 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,914 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,917 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,917 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,919 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,920 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,927 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,927 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,929 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,929 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,932 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,933 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,971 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,972 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,975 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,975 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:18,978 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,979 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,995 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,995 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:18,997 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:18,998 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,001 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,001 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,021 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,022 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,023 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,023 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,025 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,025 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,053 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,053 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,078 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,078 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,083 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,083 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,247 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,248 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,249 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,249 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,250 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,250 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,263 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,263 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,264 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,264 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,266 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,266 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,281 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,281 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,282 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,283 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,284 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,284 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,289 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,289 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,290 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,291 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,292 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,292 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,305 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,306 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,307 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,307 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,309 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,309 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,332 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,332 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,333 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,334 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,336 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,336 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,345 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,345 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,347 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,347 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,349 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,349 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,357 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,357 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,358 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,359 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,360 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,361 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,368 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,368 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,369 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,369 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,371 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,371 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,399 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,399 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,400 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,400 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,401 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,401 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,406 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,406 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,407 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,407 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,408 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,408 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,418 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,418 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,419 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,419 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,420 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,420 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,431 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,432 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,432 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,432 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,433 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,433 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,454 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,454 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,455 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,455 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,456 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,456 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,465 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,465 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,466 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,466 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,467 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,467 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,473 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,473 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,474 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,474 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,475 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,475 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,498 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,499 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,499 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,500 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,500 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,501 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,510 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,510 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,511 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,511 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,512 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,512 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,548 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,549 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,549 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,549 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,550 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,551 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,554 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,554 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,555 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,555 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,556 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,556 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,565 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,565 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,566 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,566 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,567 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,567 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,577 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,577 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,578 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,578 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,579 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,579 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,600 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,600 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,601 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,601 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,602 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,602 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,611 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,611 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,612 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,612 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,612 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,613 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,618 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,618 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,619 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,619 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,620 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,620 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,643 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,643 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,643 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,644 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,645 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,645 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,654 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,654 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,655 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,655 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,656 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,656 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,686 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,686 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,687 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,687 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,688 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,688 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,692 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,692 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,693 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,693 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,694 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,694 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,704 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,704 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,705 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,705 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,706 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,706 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,718 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,718 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,719 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,719 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,720 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,720 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,742 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,743 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,743 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,743 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,744 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,745 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,753 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,753 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,754 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,754 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,755 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,755 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,761 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,761 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,762 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,762 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,763 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,763 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,788 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,788 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,788 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,789 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,789 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,790 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,798 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,798 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,799 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,799 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,800 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,800 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,844 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,845 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,845 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,846 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,847 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,847 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,851 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,851 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,851 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,852 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,852 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,853 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,862 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,862 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,863 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,863 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,864 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,864 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,878 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,878 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,879 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,880 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,881 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,881 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,912 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,913 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,914 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,914 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,915 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,915 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,927 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,927 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,928 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,928 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:19,930 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:19,930 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:19,938 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,939 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,940 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,940 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,941 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,941 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,976 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,977 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,977 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,978 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,979 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,979 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,990 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,990 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:19,991 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,991 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:19,992 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:19,993 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,085 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,086 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,086 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,087 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,087 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,088 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,092 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,092 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,092 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,092 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,093 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,093 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,102 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,103 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,103 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,103 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,105 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,105 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,117 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,118 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,118 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,119 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,120 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,120 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,142 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,142 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,143 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,143 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,144 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,144 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,153 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,153 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,153 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,153 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,154 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,155 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,160 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,160 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,161 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,161 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,162 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,162 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,185 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,185 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,185 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,186 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,186 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,187 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,195 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,196 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,196 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,196 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,197 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,197 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,232 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,232 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,233 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,233 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,234 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,234 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,238 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,238 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,238 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,239 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,239 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,240 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,248 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,248 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,249 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,249 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,250 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,250 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,261 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,261 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,262 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,262 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,263 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,263 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,284 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,285 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,285 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,285 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,286 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,286 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,295 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,295 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,296 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,296 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,297 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,297 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,302 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,303 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,303 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,303 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,304 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,304 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,326 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,326 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,326 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,327 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,327 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,328 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,336 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,336 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,337 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,337 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,338 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,338 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,366 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,367 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,368 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,368 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,369 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,369 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,374 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,375 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,376 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,376 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,377 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,377 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,392 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,392 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,393 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,393 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,395 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,395 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,413 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,413 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,415 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,415 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,416 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,416 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,452 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,452 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,454 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,454 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,455 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,455 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,467 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,467 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,468 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,468 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,469 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,469 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,476 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,476 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,476 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,477 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,478 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,478 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,502 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,503 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,503 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,503 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,504 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,504 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,514 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,514 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,515 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,515 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,516 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,516 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,562 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,562 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,563 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,563 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,564 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,564 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,568 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,568 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,569 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,569 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,570 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,570 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,579 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,579 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,580 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,580 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,581 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,581 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,592 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,592 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,592 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,592 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,593 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,593 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,615 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,615 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,616 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,616 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,617 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,617 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,625 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,625 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,626 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,626 matplotlib.ticker [DEBUG] - ticklocs array([2.e+03, 3.e+03, 4.e+03, 5.e+03, 6.e+03, 7.e+03, 8.e+03, 9.e+03,
2.e+04, 3.e+04, 4.e+04, 5.e+04, 6.e+04, 7.e+04, 8.e+04, 9.e+04,
2.e+05, 3.e+05, 4.e+05, 5.e+05, 6.e+05, 7.e+05, 8.e+05, 9.e+05,
2.e+06, 3.e+06, 4.e+06, 5.e+06, 6.e+06, 7.e+06, 8.e+06, 9.e+06,
2.e+07, 3.e+07, 4.e+07, 5.e+07, 6.e+07, 7.e+07, 8.e+07, 9.e+07,
2.e+08, 3.e+08, 4.e+08, 5.e+08, 6.e+08, 7.e+08, 8.e+08, 9.e+08,
2.e+09, 3.e+09, 4.e+09, 5.e+09, 6.e+09, 7.e+09, 8.e+09, 9.e+09,
2.e+10, 3.e+10, 4.e+10, 5.e+10, 6.e+10, 7.e+10, 8.e+10, 9.e+10,
2.e+11, 3.e+11, 4.e+11, 5.e+11, 6.e+11, 7.e+11, 8.e+11, 9.e+11])
2023-09-16 12:25:20,627 matplotlib.ticker [DEBUG] - vmin 77935.87748881834 vmax 7223245205.6766815
2023-09-16 12:25:20,627 matplotlib.ticker [DEBUG] - ticklocs array([1.e+03, 1.e+04, 1.e+05, 1.e+06, 1.e+07, 1.e+08, 1.e+09, 1.e+10,
1.e+11])
2023-09-16 12:25:20,634 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,634 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,635 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,635 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,636 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,636 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,671 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,671 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,673 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,673 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,675 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,675 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,690 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,691 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
2023-09-16 12:25:20,692 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,692 matplotlib.ticker [DEBUG] - ticklocs array([2.e-07, 3.e-07, 4.e-07, 5.e-07, 6.e-07, 7.e-07, 8.e-07, 9.e-07,
2.e-06, 3.e-06, 4.e-06, 5.e-06, 6.e-06, 7.e-06, 8.e-06, 9.e-06,
2.e-05, 3.e-05, 4.e-05, 5.e-05, 6.e-05, 7.e-05, 8.e-05, 9.e-05,
2.e-04, 3.e-04, 4.e-04, 5.e-04, 6.e-04, 7.e-04, 8.e-04, 9.e-04,
2.e-03, 3.e-03, 4.e-03, 5.e-03, 6.e-03, 7.e-03, 8.e-03, 9.e-03,
2.e-02, 3.e-02, 4.e-02, 5.e-02, 6.e-02, 7.e-02, 8.e-02, 9.e-02,
2.e-01, 3.e-01, 4.e-01, 5.e-01, 6.e-01, 7.e-01, 8.e-01, 9.e-01,
2.e+00, 3.e+00, 4.e+00, 5.e+00, 6.e+00, 7.e+00, 8.e+00, 9.e+00,
2.e+01, 3.e+01, 4.e+01, 5.e+01, 6.e+01, 7.e+01, 8.e+01, 9.e+01,
2.e+02, 3.e+02, 4.e+02, 5.e+02, 6.e+02, 7.e+02, 8.e+02, 9.e+02])
2023-09-16 12:25:20,693 matplotlib.ticker [DEBUG] - vmin 7.82714747784675e-06 vmax 1.295687196987124
2023-09-16 12:25:20,694 matplotlib.ticker [DEBUG] - ticklocs array([1.e-07, 1.e-06, 1.e-05, 1.e-04, 1.e-03, 1.e-02, 1.e-01, 1.e+00,
1.e+01, 1.e+02])
Total running time of the script: (2 minutes 21.760 seconds)